JavaScript: Find the maximum difference among all possible pairs of a given array of integers
JavaScript Basic: Exercise-93 with Solution
Find Max Difference Among All Pairs in Array
Write a JavaScript program to find the maximum difference among all possible pairs of a given array of integers.
Sample Solution:
JavaScript Code:
// Function to find the maximum difference between any two elements in an array
function array_max_diff(arr) {
var max_result = 0; // Initialize the variable to store the maximum difference
// Iterate through the array elements
for(var i = 0; i < arr.length; i++) {
// Iterate through other elements in the array
for(var k = 0; k !== i && k < arr.length; k++) {
var diff = Math.abs(arr[i] - arr[k]); // Calculate the absolute difference
max_result = Math.max(max_result, diff); // Update the maximum difference
}
}
return max_result; // Return the final maximum difference
}
// Example usage
console.log(array_max_diff([1, 2, 3, 8, 9])); // 8
console.log(array_max_diff([1, 2, 3, 18, 9])); // 17
console.log(array_max_diff([13, 2, 3, 8, 9])); // 11
Output:
8 17 11
Live Demo:
See the Pen javascript-basic-exercise-93 by w3resource (@w3resource) on CodePen.
Flowchart:
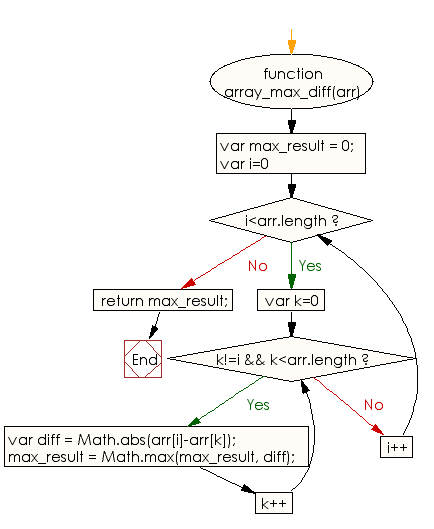
ES6 Version:
// Function to find the maximum difference between elements in an array
const array_max_diff = (arr) => {
let max_result = 0; // Initialize the maximum difference variable
// Iterate through the array to calculate the differences between elements
for (let i = 0; i < arr.length; i++) {
for (let k = 0; k !== i && k < arr.length; k++) {
const diff = Math.abs(arr[i] - arr[k]); // Calculate the absolute difference
max_result = Math.max(max_result, diff); // Update the maximum difference
}
}
return max_result; // Return the final maximum difference
};
// Example usage
console.log(array_max_diff([1, 2, 3, 8, 9])); // 8
console.log(array_max_diff([1, 2, 3, 18, 9])); // 17
console.log(array_max_diff([13, 2, 3, 8, 9])); // 11
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the maximum difference among all possible pairs in an array by computing the difference between the minimum and maximum values.
- Write a JavaScript function that iterates over an array only once to determine the overall maximum and minimum, then calculates their difference.
- Write a JavaScript program that efficiently computes the maximum absolute difference between any two elements in an array without nested loops.
Go to:
PREV : Find Max Difference Between Adjacent Elements.
NEXT : Find Most Frequent Number in Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.