JavaScript: Find the maximum difference between any two adjacent elements of a given array of integers
JavaScript Basic: Exercise-92 with Solution
Write a JavaScript program to find the maximum difference between any two adjacent elements of a given array of integers.
Visual Presentation:
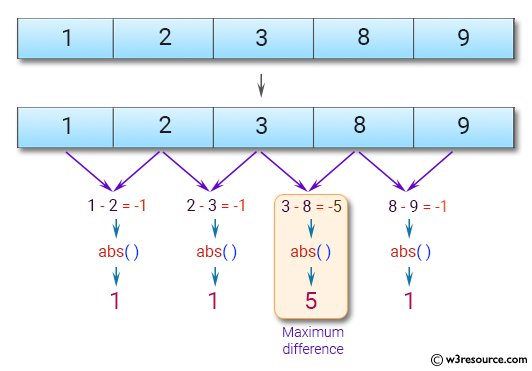
Sample Solution:
JavaScript Code:
// Function to find the maximum difference between adjacent elements in an array
function max_difference(arr) {
var max = -1; // Initialize a variable to store the maximum difference
var temp; // Temporary variable to calculate the difference
// Iterate through the array to find the maximum difference
for (var i = 0; i < arr.length - 1; i++) {
temp = Math.abs(arr[i] - arr[i + 1]); // Calculate the absolute difference
max = Math.max(max, temp); // Update the maximum difference
}
return max; // Return the final maximum difference
}
// Example usage
console.log(max_difference([1, 2, 3, 8, 9])); // 5
console.log(max_difference([1, 2, 3, 18, 9])); // 15
console.log(max_difference([13, 2, 3, 8, 9])); // 11
Output:
5 15 11
Live Demo:
See the Pen javascript-basic-exercise-92 by w3resource (@w3resource) on CodePen.
Flowchart:
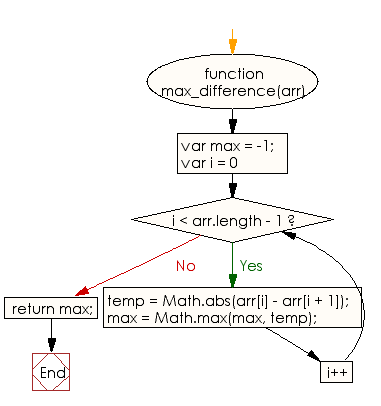
ES6 Version:
// Function to find the maximum difference between consecutive elements in an array
const max_difference = (arr) => {
let max = -1; // Initialize the maximum difference variable with -1
let temp; // Temporary variable to store the absolute difference
// Iterate through the array to calculate the differences between consecutive elements
for (let i = 0; i < arr.length - 1; i++) {
temp = Math.abs(arr[i] - arr[i + 1]); // Calculate the absolute difference
max = Math.max(max, temp); // Update the maximum difference
}
return max; // Return the final maximum difference
};
// Example usage
console.log(max_difference([1, 2, 3, 8, 9])); // 5
console.log(max_difference([1, 2, 3, 18, 9])); // 15
console.log(max_difference([13, 2, 3, 8, 9])); // 11
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the maximum possible sum of some of its k consecutive numbers (numbers that follow each other in order.) of a given array of positive integers.
Next: JavaScript program to find the maximum difference among all possible pairs of a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics