JavaScript: Find the maximum possible sum of some of its k consecutive numbers of a specified array of positive integers
JavaScript Basic: Exercise-91 with Solution
Find Max Sum of k Consecutive Numbers in Array
Write a JavaScript program to find the maximum possible sum of some of its k consecutive numbers (numbers that follow each other in order) in a given array of positive integers.
Visual Presentation:
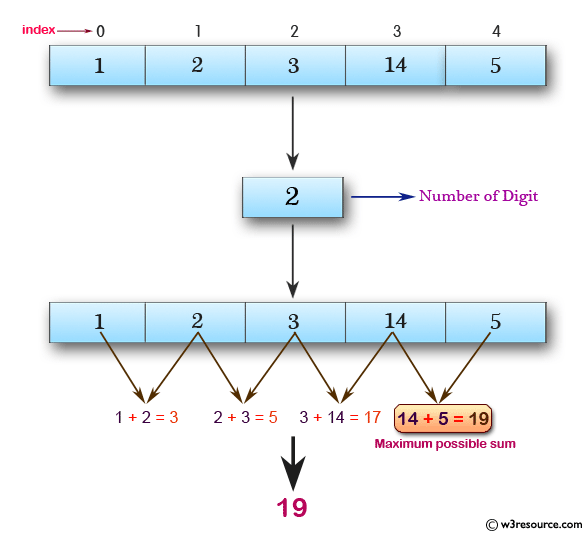
Sample Solution:
JavaScript Code:
function array_max_consecutive_sum(nums, k) {
let result = 0;
let temp_sum = 0;
for (var i = 0; i < k - 1; i++) {
temp_sum += nums[i];
}
for (var i = k - 1; i < nums.length; i++) {
temp_sum += nums[i];
if (temp_sum > result) {
result = temp_sum;
}
temp_sum -= nums[i - k + 1];
}
return result;
}
console.log(array_max_consecutive_sum([1, 2, 3, 14, 5], 2))
console.log(array_max_consecutive_sum([2, 3, 5, 1, 6], 3))
console.log(array_max_consecutive_sum([9, 3, 5, 1, 7], 2))
Output:
19 12 12
Live Demo:
See the Pen javascript-basic-exercise-91 by w3resource (@w3resource) on CodePen.
Flowchart:
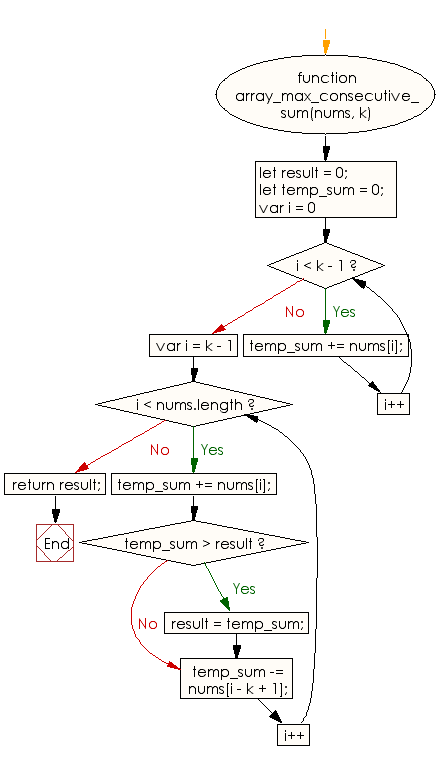
ES6 Version:
// Function to find the maximum consecutive sum of k elements in an array
const array_max_consecutive_sum = (nums, k) => {
let result = 0; // Initialize the result variable
let temp_sum = 0; // Initialize a variable to store the temporary sum
// Calculate the initial temporary sum for the first k-1 elements
for (let i = 0; i < k - 1; i++) {
temp_sum += nums[i];
}
// Iterate through the array, updating the temporary sum and result
for (let i = k - 1; i < nums.length; i++) {
temp_sum += nums[i];
// Update the result if the temporary sum is greater
if (temp_sum > result) {
result = temp_sum;
}
// Subtract the first element of the current k elements from the temporary sum
temp_sum -= nums[i - k + 1];
}
// Return the final result
return result;
};
// Example usage
console.log(array_max_consecutive_sum([1, 2, 3, 14, 5], 2));
console.log(array_max_consecutive_sum([2, 3, 5, 1, 6], 3));
console.log(array_max_consecutive_sum([9, 3, 5, 1, 7], 2));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that uses a sliding window technique to find the maximum sum of k consecutive elements in an array.
- Write a JavaScript function that iterates over an array with a fixed-size window to compute and return the maximum possible sum.
- Write a JavaScript program that validates the value of k against the array length and then efficiently calculates the maximum sum of k consecutive numbers.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the kth greatest element of a given array of integers.
Next: JavaScript program to find the maximum difference between any two adjacent elements of a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.