JavaScript: Check whether it is possible to replace $ in a specified expression
JavaScript Basic: Exercise-89 with Solution
Replace $ in Expression to Make True
Write a JavaScript program to check whether it is possible to replace $ in a given expression x $ y = z with one of the four signs +, -, * or / to obtain a correct expression.
For example x = 10, y = 30 and z = 300, we can replace $ with a multiple operator (*) to obtain x * y = z
Visual Presentation:
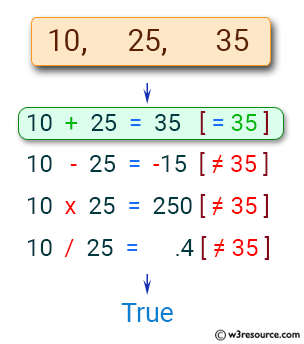
Sample Solution:
JavaScript Code:
// Function to check if there is an arithmetic expression (addition, multiplication, division, or subtraction) among three numbers
function check_arithmetic_Expression(x, y, z) {
// Check if any of the arithmetic expressions holds true
return x + y == z || x * y == z || x / y == z || x - y == z;
}
// Examples of using the function
console.log(check_arithmetic_Expression(10, 25, 35)); // true, as 10 + 25 = 35
console.log(check_arithmetic_Expression(10, 25, 250)); // true, as 10 * 25 = 250
console.log(check_arithmetic_Expression(30, 25, 5)); // true, as 30 / 25 = 1.2
console.log(check_arithmetic_Expression(100, 25, 4.0)); // true, as 100 / 25 = 4.0
console.log(check_arithmetic_Expression(100, 25, 25)); // true, as 100 - 25 = 75
Output:
true true true true false
Live Demo:
See the Pen javascript-basic-exercise-89 by w3resource (@w3resource) on CodePen.
Flowchart:
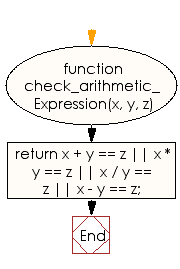
ES6 Version:
// Function to check if any of the arithmetic expressions (addition, multiplication, division, subtraction) is true
const check_arithmetic_Expression = (x, y, z) => {
// Return true if any of the arithmetic expressions is true
return x + y == z || x * y == z || x / y == z || x - y == z;
};
// Example usage
console.log(check_arithmetic_Expression(10, 25, 35)); // true
console.log(check_arithmetic_Expression(10, 25, 250)); // true
console.log(check_arithmetic_Expression(30, 25, 5)); // true
console.log(check_arithmetic_Expression(100, 25, 4.0)); // true
console.log(check_arithmetic_Expression(100, 25, 25)); // false
For more Practice: Solve these Related Problems:
- Write a JavaScript program that takes an expression containing a '$' operator and tests each arithmetic operator (+, -, *, /) to see which one validates the equation.
- Write a JavaScript function that iterates over the four arithmetic operators to replace '$' in a given expression and returns the valid operator if found.
- Write a JavaScript program that parses a string expression with a placeholder and systematically checks all operations to determine which one makes the equation true, while handling division by zero.
Go to:
PREV : Check Similarity of Two Integers with Divisor.
NEXT : Find kth Greatest Element in Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.