JavaScript: Check whether two arrays of integers of same length are similar or not
JavaScript Basic: Exercise-87 with Solution
Check if Two Arrays Are Similar with One Swap
Write a JavaScript program to determine if two arrays of integers of the same length are similar. The arrays will be similar if one array can be obtained from another array by swapping at most one pair of elements.
Visual Presentation:
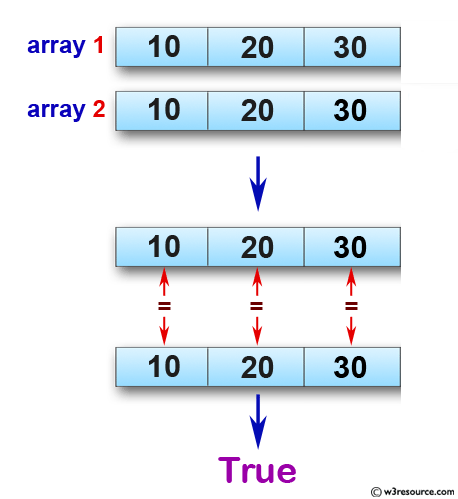
Sample Solution:
JavaScript Code:
// Function to check if rearranging elements of one array can make it equal to another array
function array_checking(arra1, arra2) {
// Iterate through elements of arra1
for (var i = 0; i < arra1.length; i++) {
// Nested loop to swap elements and check permutations
for (var j = i; j < arra1.length; j++) {
// Initialize result and temporary variable
var result = true,
temp = arra1[i];
// Swap elements
arra1[i] = arra1[j];
arra1[j] = temp;
// Compare elements of the modified arra1 with arra2
for (var k = 0; k < arra1.length; k++) {
if (arra1[k] !== arra2[k]) {
result = false;
break;
}
}
// If the arrays match, return true
if (result) {
return true;
}
// Restore the original order by swapping back elements
arra1[j] = arra1[i];
arra1[i] = temp;
}
}
// If no match is found, return false
return false;
}
// Example usage
console.log(array_checking([10, 20, 30], [10, 20, 30])); // true
console.log(array_checking([10, 20, 30], [30, 10, 20])); // true
console.log(array_checking([10, 20, 30, 40], [10, 30, 20, 40])); // false
Output:
true false true
Live Demo:
See the Pen javascript-basic-exercise-87 by w3resource (@w3resource) on CodePen.
Flowchart:
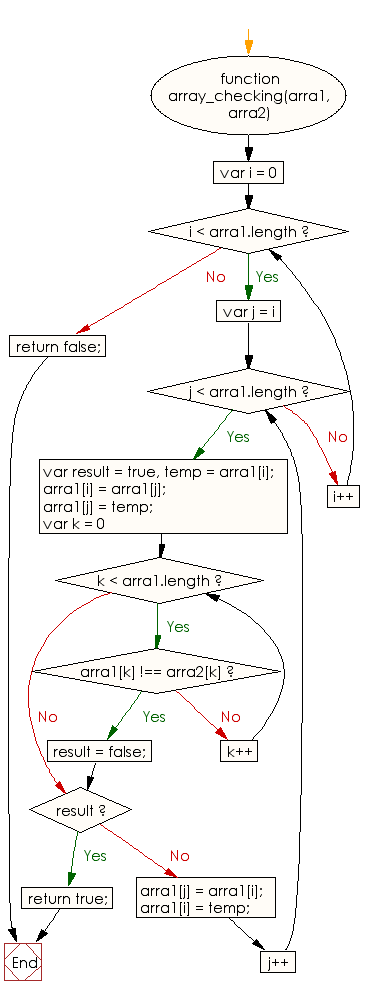
ES6 Version:
// Function to check if two arrays are equal regardless of the order of elements
const array_checking = (arra1, arra2) => {
// Loop through the elements of the first array
for (let i = 0; i < arra1.length; i++) {
// Nested loop starting from i to compare different combinations of elements
for (let j = i; j < arra1.length; j++) {
// Swap elements to create different combinations
let result = true,
temp = arra1[i];
arra1[i] = arra1[j];
arra1[j] = temp;
// Check if the current combination is equal to the second array
for (let k = 0; k < arra1.length; k++) {
if (arra1[k] !== arra2[k]) {
result = false;
break;
}
}
// If the combination matches, return true
if (result) {
return true;
}
// Swap elements back to their original positions
arra1[j] = arra1[i];
arra1[i] = temp;
}
}
// If no matching combination is found, return false
return false;
};
// Example usage
console.log(array_checking([10, 20, 30], [10, 20, 30])); // true
console.log(array_checking([10, 20, 30], [30, 10, 20])); // true
console.log(array_checking([10, 20, 30, 40], [10, 30, 20, 40])); // false
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the types of a given array.
Next: JavaScript program that takes two integers and a divisor. If the given divisor divides both integers and it does not divide either, then two given integers are similar. Check whether two given integers are similar or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics