JavaScript: Replace each character of a given string by the next one in the English alphabet
JavaScript Basic: Exercise-84 with Solution
Replace Characters with Next in Alphabet
Write a JavaScript program to replace each character in a given string with the next in the English alphabet.
Note: ‘a’ will be replace by ‘b’ or ‘z’ would be replaced by ‘a’.
Visual Presentation:
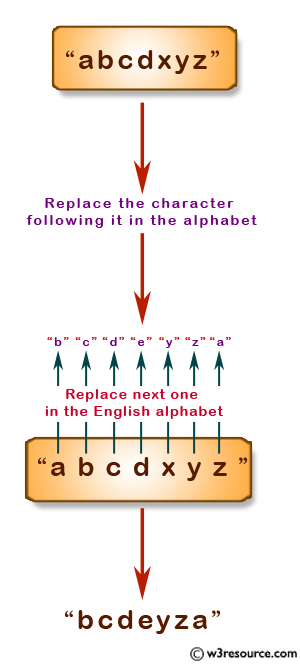
Sample Solution:
JavaScript Code:
Output:
bcdeyza
Explanation:
In the exercise above,
- The input string 'str' is split into an array of individual characters using the "split()" method and stored in the variable 'all_chars'.
- A "for" loop iterates over each character in the 'all_chars' array.
- For each character,
- Its Unicode value is obtained using the "charCodeAt()" method, and the Unicode value of 'a' is subtracted to determine its position in the alphabet.
- The position is shifted to the next character in the alphabet using modular arithmetic ((n + 1) % 26). This ensures that if the character is 'z', it wraps around to 'a'.
- The shifted position is converted back to the corresponding character using "String.fromCharCode()" and 'a''s Unicode value is added back.
- The original character in the array is replaced with the shifted character.
- Finally, the "join()" method is used to join the array of characters back into a single string.
Live Demo:
Flowchart:
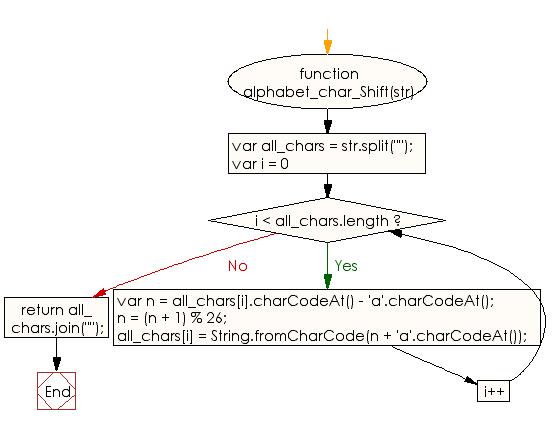
ES6 Version:
For more Practice: Solve these Related Problems:
- Write a JavaScript program that replaces each letter in a string with the next letter in the alphabet, wrapping 'z' to 'a' and preserving case.
- Write a JavaScript function that shifts every alphabetic character forward by one, leaving non-letter characters unchanged.
- Write a JavaScript program that transforms a string by incrementing each letter, using char codes and handling edge cases like 'Z' and 'z'.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript to find the longest string from an given array of strings.
Next: JavaScript code to divide an given array of positive integers into two parts.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.