JavaScript: Find the longest string from a given array of strings
JavaScript Basic: Exercise-83 with Solution
Find Longest String in Array
Write a JavaScript program to find the longest string from a given array of strings.
Visual Presentation:
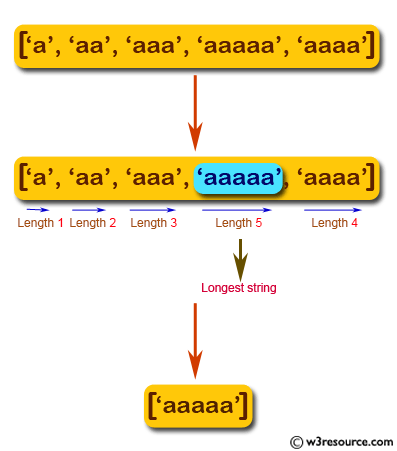
Sample Solution:
JavaScript Code:
// Function to find the longest string(s) in an array
function longest_string(str_ara) {
var max = str_ara[0].length; // Initialize max length with the length of the first string
str_ara.map(v => max = Math.max(max, v.length)); // Update max with the maximum length in the array using the map function
result = str_ara.filter(v => v.length == max); // Filter out strings that have the maximum length
return result; // Return the array of longest strings
}
// Example usage
console.log(longest_string(['a', 'aa', 'aaa', 'aaaaa', 'aaaa']));
Output:
["aaaaa"]
Live Demo:
See the Pen javascript-basic-exercise-83 by w3resource (@w3resource) on CodePen.
Flowchart:
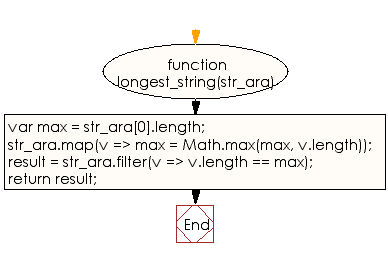
ES6 Version:
// ES6 version with comments
// Function to find the longest strings in an array
const longest_string = (str_ara) => {
let max = str_ara[0].length; // Initialize max length with the length of the first string
str_ara.map(v => max = Math.max(max, v.length)); // Update max by finding the maximum length in the array
result = str_ara.filter(v => v.length == max); // Filter strings that have the maximum length
return result; // Return the result array
};
// Example usage
console.log(longest_string(['a', 'aa', 'aaa', 'aaaaa', 'aaaa']));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that iterates through an array of strings and returns the longest one, handling ties by choosing the first occurrence.
- Write a JavaScript function that uses the reduce() method to find and return the longest string in an array.
- Write a JavaScript program that checks an array of strings for the one with maximum length and outputs it, accounting for empty arrays.
Go to:
PREV : Add Two Integers Without Carrying.
NEXT : Replace Characters with Next in Alphabet.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.