JavaScript: Add two positive integers without carry
JavaScript Basic: Exercise-82 with Solution
Add Two Integers Without Carrying
Write a JavaScript program to add two positive integers without carrying.
Visual Presentation:
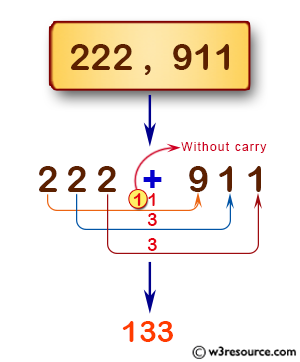
Sample Solution:
JavaScript Code:
// Function to add two integers without carrying
function add_two_int_without_carrying(n1, n2) {
var result = 0, // Initialize the result
x = 1; // Initialize the multiplier
// Iterate while both numbers have digits
while (n1 > 0 && n2 > 0) {
result += x * ((n1 + n2) % 10); // Add the current digit without carrying
n1 = Math.floor(n1 / 10); // Move to the next digit in the first number
n2 = Math.floor(n2 / 10); // Move to the next digit in the second number
x *= 10; // Update the multiplier for the next digit place
}
return result; // Return the final result
}
// Example usage
console.log(add_two_int_without_carrying(222, 911)); // Output: 133
console.log(add_two_int_without_carrying(200, 900)); // Output: 100
Output:
133 100
Live Demo:
See the Pen javascript-basic-exercise-82 by w3resource (@w3resource) on CodePen.
Flowchart:
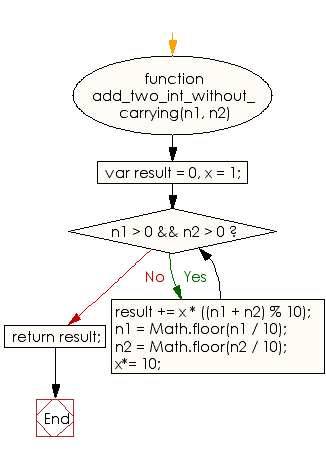
ES6 Version:
// ES6 version with comments
// Function to add two integers without carrying
const add_two_int_without_carrying = (n1, n2) => {
let result = 0; // Initialize the result
let x = 1; // Initialize the place value
// Continue until both numbers become zero
while (n1 > 0 && n2 > 0) {
// Add the sum of the last digits multiplied by the current place value to the result
result += x * ((n1 + n2) % 10);
// Update n1 and n2 by removing the last digit
n1 = Math.floor(n1 / 10);
n2 = Math.floor(n2 / 10);
// Update the place value by multiplying it by 10
x *= 10;
}
// Return the final result
return result;
};
// Example usage
console.log(add_two_int_without_carrying(222, 911));
console.log(add_two_int_without_carrying(200, 900));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that adds two positive integers without carrying over, processing each digit independently.
- Write a JavaScript function that simulates addition without carrying by converting numbers to strings and summing corresponding digits.
- Write a JavaScript program that performs digit-by-digit addition without carrying and returns the final computed result.
Go to:
PREV : Add Two Digits in a Two-Digit Number.
NEXT : Find Longest String in Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.