JavaScript: Add two digits of a given positive integer of length two
JavaScript Basic: Exercise-81 with Solution
Write a JavaScript program to add two digits to a given positive integer of length two.
Visual Presentation:
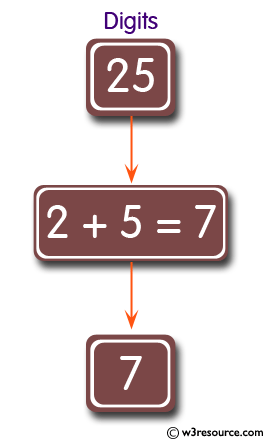
Sample Solution:
JavaScript Code:
// Function to add the two digits of a number
function add_two_digits(n) {
// Return the sum of the last digit and the result of dividing the number by 10 and rounding down
return n % 10 + Math.floor(n / 10);
}
// Example usage
console.log(add_two_digits(25)); // Output: 7 (2 + 5)
console.log(add_two_digits(50)); // Output: 5 (5 + 0)
Output:
7 5
Live Demo:
See the Pen javascript-basic-exercise-81 by w3resource (@w3resource) on CodePen.
Flowchart:
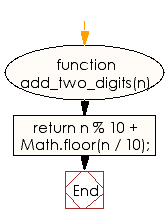
ES6 Version:
// Defining a function 'add_two_digits' that takes a number 'n' as an argument
const add_two_digits = (n) => {
// Returning the sum of the last digit (n % 10) and the digit in the tens place (Math.floor(n / 10))
return n % 10 + Math.floor(n / 10);
};
// Printing the result of calling the function 'add_two_digits' with the arguments 25 and 50
console.log(add_two_digits(25));
console.log(add_two_digits(50));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to swap the first and last elements of a given array of integers. The array length should be at least 1.
Next: JavaScript to add two positive integers without carry.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics