JavaScript: Find the larger value between the first or last and set all the other elements with that value
JavaScript Basic: Exercise-74 with Solution
Set All Elements to Largest of First/Last in Array
Write a JavaScript program to find the largest value between the first and last elements and set all the other elements to that value. Display the updated array.
The program compares the first and last elements of an array and sets all other elements to the larger of these two values. It then displays the updated array with all elements either equal to the first or last element, whichever is larger.
Visual Presentation:
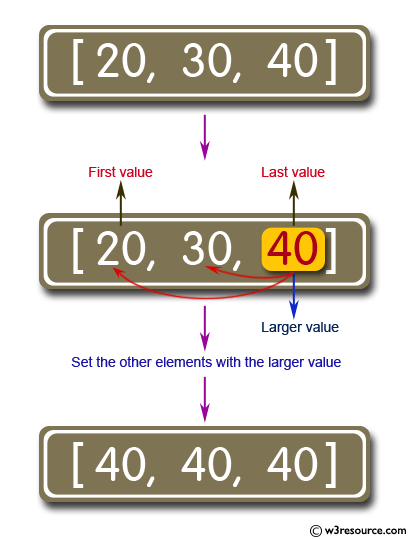
Sample Solution:
JavaScript Code:
// Define a function named all_max that takes an array of numbers as a parameter
function all_max(nums)
{
// Determine the maximum value between the first and third elements using a ternary operator
var max_val = nums[0] > nums[2] ? nums[0] : nums[2];
// Set all elements in the array to the determined maximum value
nums[0] = max_val;
nums[1] = max_val;
nums[2] = max_val;
// Return the modified array
return nums;
}
// Call the function with sample arguments and log the results to the console
console.log(all_max([20, 30, 40])); // Output: [40, 40, 40]
console.log(all_max([-7, -9, 0])); // Output: [0, 0, 0]
console.log(all_max([12, 10, 3])); // Output: [12, 12, 12]
Output:
[40,40,40] [0,0,0] [12,12,12]
Live Demo:
See the Pen JavaScript - Find the larger value between the first or last - basic-ex-74 by w3resource (@w3resource) on CodePen.
Flowchart:
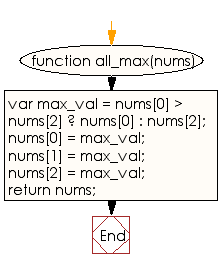
ES6 Version:
// Define a function named all_max that takes an array (nums) as a parameter
const all_max = (nums) => {
// Use the spread operator to create a copy of the array, then find the maximum value
const max_val = Math.max(...nums);
// Update all elements of the array with the maximum value
nums[0] = max_val;
nums[1] = max_val;
nums[2] = max_val;
// Return the modified array
return nums;
};
// Call the function with sample arguments and log the results to the console
console.log(all_max([20, 30, 40])); // Output: [40, 40, 40]
console.log(all_max([-7, -9, 0])); // Output: [0, 0, 0]
console.log(all_max([12, 10, 3])); // Output: [12, 12, 12]
For more Practice: Solve these Related Problems:
- Write a JavaScript program that identifies the larger of the first and last elements in an array and then sets every element of the array to that value.
- Write a JavaScript function that updates an array by replacing all its elements with the maximum value found between the first and last items.
- Write a JavaScript program that validates an array, determines the greater value between its boundaries, and overwrites all elements with that value.
Go to:
PREV : Reverse Elements of Array (Length 3).
NEXT : Create Array with Middle Elements from Two Arrays.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.