JavaScript: Rotate the elements left of a given array of integers of length 3
JavaScript Basic: Exercise-70 with Solution
Rotate Elements Left in Array (Length 3)
Write a JavaScript program to rotate the elements left in a given array of integers of length 3.
The program takes an array of three integers and rotates its elements to the left, meaning the first element moves to the end, the second element moves to the first position, and the third element moves to the second position. The modified array is then returned or displayed.
Visual Presentation:
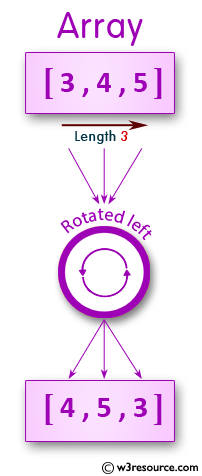
Sample Solution:
JavaScript Code:
// Define a function named rotate_elements_left with a parameter array
function rotate_elements_left(array)
{
// Return a new array with elements rotated to the left
// The new order is [array[1], array[2], array[0]]
return [array[1], array[2], array[0]];
}
// Call the function with sample arguments and log the results to the console
console.log(rotate_elements_left([3, 4, 5]));
console.log(rotate_elements_left([0, -1, 2]));
console.log(rotate_elements_left([7, 6, 5]));
Output:
[4,5,3] [-1,2,0] [6,5,7]
Live Demo:
See the Pen JavaScript - Rotate the elements left of a given array of integers of length 3 - basic-ex-70 by w3resource (@w3resource) on CodePen.
Flowchart:
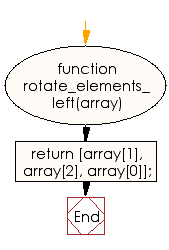
ES6 Version:
// Define a function named rotate_elements_left with a parameter array using arrow function syntax
const rotate_elements_left = array => {
// Use array destructuring to rearrange elements
const [second, third, first] = array;
// Return a new array with elements rearranged
return [second, third, first];
};
// Call the function with sample arguments and log the results to the console
console.log(rotate_elements_left([3, 4, 5]));
console.log(rotate_elements_left([0, -1, 2]));
console.log(rotate_elements_left([7, 6, 5]));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that rotates the elements of a three-element array to the left, moving the first element to the end.
- Write a JavaScript function that cyclically shifts a length-3 array to the left without using built-in rotation functions.
- Write a JavaScript program that verifies an array has exactly three elements before performing a left rotation and returning the new array.
Go to:
PREV : Sum of 3 Elements in Array (Length 3).
NEXT : Check if 1 is First/Last Element in Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.