JavaScript: Compute the sum of three elements of a given array of integers of length 3
JavaScript Basic: Exercise-69 with Solution
Sum of 3 Elements in Array (Length 3)
Write a JavaScript program to compute the sum of three elements of a given array of integers of length 3.
The program takes an array of three integers as input and calculates the sum of these three elements. It then returns or displays the resulting sum.
Visual Presentation:
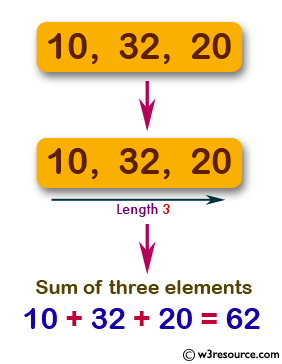
Sample Solution:
JavaScript Code:
// Define a function named sum_three that takes an array of three numbers as a parameter
function sum_three(nums) {
// Return the sum of the three numbers using array indexing
return nums[0] + nums[1] + nums[2];
}
// Call the function with different arrays and log the results to the console
console.log(sum_three([10, 32, 20]));
console.log(sum_three([5, 7, 9]));
console.log(sum_three([0, 8, -11]));
Output:
62 21 -3
Live Demo:
See the Pen JavaScript - compute the sum of three elements of a given array of integers of length 3 - basic-ex-69 by w3resource (@w3resource) on CodePen.
Flowchart:
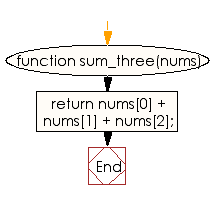
ES6 Version:
// Define a function named sum_three with a parameter nums using arrow function syntax
const sum_three = nums => {
// Use destructuring assignment to get individual elements of the array
const [num1, num2, num3] = nums;
// Return the sum of the three numbers
return num1 + num2 + num3;
};
// Call the function with sample arguments and log the results to the console
console.log(sum_three([10, 32, 20]));
console.log(sum_three([5, 7, 9]));
console.log(sum_three([0, 8, -11]));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create a new string taking the first and last n characters from a given string.
Next: JavaScript program to rotate the elements left of a given array of integers of length 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics