JavaScript: Create a new string taking the first and last n characters from a given string
JavaScript Basic: Exercise-68 with Solution
Use First and Last 'n' Characters from String
Write a JavaScript program to create a new string using the first and last n characters from a given string. The string length must be larger than or equal to n.
The program takes a string and an integer n, then constructs a new string using the first n characters and the last n characters from the original string. It ensures the string's length is at least n before performing this operation.
Visual Presentation:
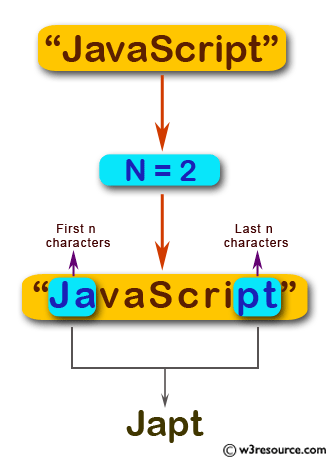
Sample Solution:
JavaScript Code:
// Define a function named two_string with parameters str and n
function two_string(str, n) {
// Extract the first part of the string from index 0 to n (exclusive)
first_part = str.substring(0, n);
// Extract the last part of the string from (length - n) to the end
last_part = str.substring(str.length - n);
// Concatenate the first and last parts and return the result
return first_part + last_part;
}
// Call the function with sample arguments and log the results to the console
console.log(two_string("JavaScript", 2));
console.log(two_string("JavaScript", 3));
Output:
Japt Javipt
Live Demo:
See the Pen Create a new string taking the first and last n characters from a given string by w3resource (@w3resource) on CodePen.
Flowchart:
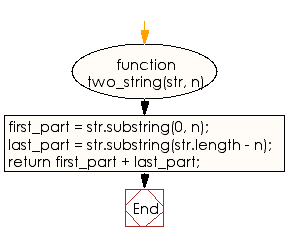
ES6 Version:
// Define a function named two_string with parameters str and n
const two_string = (str, n) => {
// Use const for constant variables and template literals for string concatenation
const first_part = str.substring(0, n);
const last_part = str.substring(str.length - n);
return `${first_part}${last_part}`;
};
// Call the function with sample arguments and log the results to the console
console.log(two_string("JavaScript", 2));
console.log(two_string("JavaScript", 3));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that takes a string and an integer n, and returns a new string made by concatenating the first n and last n characters of the original string.
- Write a JavaScript function that validates the string length is at least n before extracting and combining its starting and ending segments.
- Write a JavaScript program that dynamically creates a new string from the first and last n characters of an input string, handling invalid n values gracefully.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create a new string from a given string, removing the first and last characters of the string if the first or last character are 'P'.
Next: JavaScript program to compute the sum of three elements of a given array of integers of length 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.