JavaScript: Display the city name if the string begins with "Los" or "New" otherwise return blank
JavaScript Basic: Exercise-66 with Solution
Return City Name if Starts with 'Los' or 'New'
Write a JavaScript program to display the city name if the string begins with "Los" or "New" otherwise return blank.
Visual Presentation:
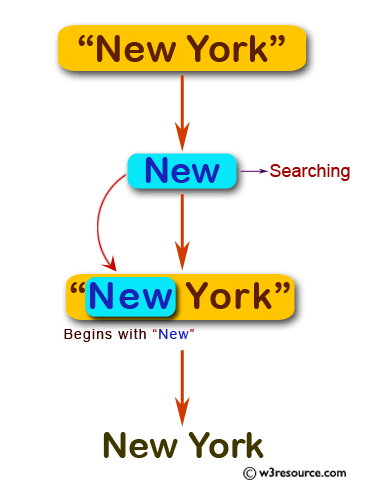
Sample Solution:
JavaScript Code:
// Define a function named city_name with parameter str
function city_name(str) {
// Check if str has length greater than or equal to 3 and starts with 'Los' or 'New'
if (str.length >= 3 && ((str.substring(0, 3) == 'Los') || (str.substring(0, 3) == 'New'))) {
// Return the input str if conditions are met
return str;
}
// Return an empty string if conditions are not met
return '';
}
// Call the function with sample arguments and log the results to the console
console.log(city_name("New York"));
console.log(city_name("Los Angeles"));
console.log(city_name("London"));
Output:
New York Los Angeles
Live Demo:
See the Pen JavaScript - Display the city name if the string begins with "Los" or "New" otherwise return blank - basic-ex-66 by w3resource (@w3resource) on CodePen.
Flowchart:
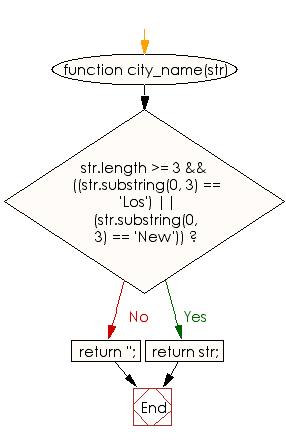
ES6 Version:
// Define a function named city_name with parameter str
const city_name = (str) => {
// Use conditional (ternary) operator to check conditions and return the result
return (str.length >= 3 && (str.substring(0, 3) === 'Los' || str.substring(0, 3) === 'New')) ? str : '';
};
// Call the function with sample arguments and log the results to the console
console.log(city_name("New York"));
console.log(city_name("Los Angeles"));
console.log(city_name("London"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that accepts a city name and returns it only if it starts with "Los" or "New", ignoring case and extra whitespace.
- Write a JavaScript function that checks the beginning of a city name and outputs it if it starts with either "Los" or "New", otherwise returns an empty string.
- Write a JavaScript program that trims and verifies a city name, returning the original name only if it begins with "Los" or "New".
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to test if a string end with "Script". The string length must be greater or equal to 6.
Next: JavaScript program to create a new string from a given string, removing the first and last characters of the string if the first or last character are 'P'.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.