JavaScript: Test whether a string end with "Script"
JavaScript Basic: Exercise-65 with Solution
Check if String Ends with 'Script'
Write a JavaScript program to test whether a string ends with "Script". The string length must be greater than or equal to 6.
The JavaScript program checks if a given string ends with "Script" and ensures the string's length is at least 6 characters. If both conditions are met, it returns true; otherwise, it returns false.
Visual Presentation:
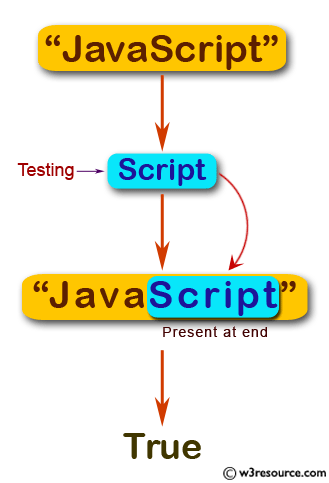
Sample Solution:
JavaScript Code:
// Define a function named end_script with parameter str
function end_script(str) {
// Check if the last 6 characters of str are equal to 'Script'
if (str.substring(str.length - 6, str.length) == 'Script') {
// Return true if the condition is met
return true;
} else {
// Return false if the condition is not met
return false;
}
}
// Call the function with sample arguments and log the results to the console
console.log(end_script("JavaScript"));
console.log(end_script("Java Script"));
console.log(end_script("Java Scripts"));
Output:
true true false
Live Demo:
See the Pen JavaScript - Test if a string end with Script - basic-ex-65 by w3resource (@w3resource) on CodePen.
Flowchart:
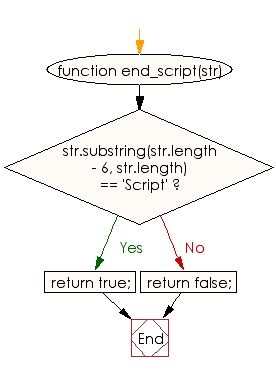
ES6 Version:
// Define a function named end_script with parameter str
const end_script = (str) => {
// Use endsWith method to check if str ends with 'Script' and return the result
return str.endsWith('Script');
};
// Call the function with sample arguments and log the results to the console
console.log(end_script("JavaScript"));
console.log(end_script("Java Script"));
console.log(end_script("Java Scripts"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if a given string of at least 6 characters ends with "Script" in a case-sensitive manner.
- Write a JavaScript function that validates a string's length and then determines if its final six characters form the word "Script".
- Write a JavaScript program that tests the ending of a string and returns a boolean indicating whether it terminates with "Script".
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to concatenate two strings and return the result.
Next: JavaScript program to display the city name if the string begins with "Los" or "New" otherwise return blank.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.