JavaScript: Create a string using the middle three characters of a given string of odd length
JavaScript Basic: Exercise-63 with Solution
Extract Middle 3 Characters from Odd-Length String
Write a JavaScript program to create a string using the middle three characters of a given string of odd length. The string length must be greater than or equal to three.
This JavaScript program extracts and creates a new string consisting of the middle three characters from an input string of odd length, ensuring the input string length is at least three.
Visual Presentation:
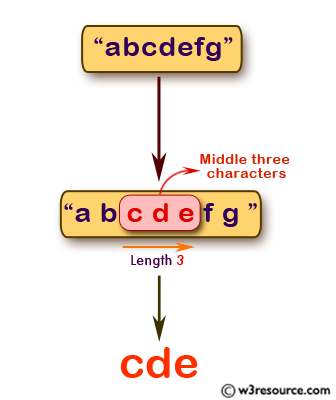
Sample Solution:
JavaScript Code:
// Define a function named middle_three with parameter str
function middle_three(str) {
// Check if the length of str is odd
if (str.length % 2 !== 0) {
// Calculate the middle index for odd-length strings
mid = (str.length + 1) / 2;
// Use slice to get the middle three characters and return
return str.slice(mid - 2, mid + 1);
}
// Return str if its length is not odd
return str;
}
// Call the function with sample arguments and log the results to the console
console.log(middle_three('abcdefg'));
console.log(middle_three('HTML5'));
console.log(middle_three('Python'));
console.log(middle_three('PHP'));
console.log(middle_three('Exercises'));
Output:
cde TML Python PHP rci
Live Demo:
See the Pen JavaScript - Create a string using the middle three characters of a given string of odd length - basic-ex-63 by w3resource (@w3resource) on CodePen.
Flowchart:
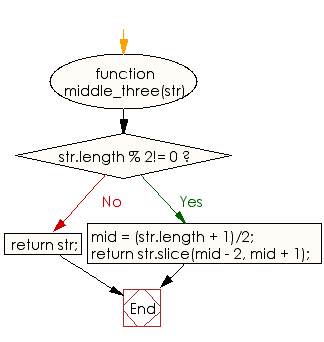
ES6 Version:
// Define a function named middle_three with parameter str
const middle_three = (str) => {
// Check if the length of str is odd
if (str.length % 2 !== 0) {
// Calculate the middle index
const mid = (str.length + 1) / 2;
// Use slice to get the middle three characters and return the result
return str.slice(mid - 2, mid + 1);
}
// Return str if its length is not odd
return str;
};
// Call the function with sample arguments and log the results to the console
console.log(middle_three('abcdefg'));
console.log(middle_three('HTML5'));
console.log(middle_three('Python'));
console.log(middle_three('PHP'));
console.log(middle_three('Exercises'));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that extracts the middle three characters from a string of odd length (length ≥ 3) using calculated indices.
- Write a JavaScript function that verifies if a string is of odd length and then returns its central three characters.
- Write a JavaScript program that calculates the center of an odd-length string and extracts a substring of three characters centered around it.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to move last three character to the start of a given string.
Next: JavaScript program to concatenate two strings and return the result.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.