JavaScript: Move last three character to the start of a specified string
JavaScript Basic: Exercise-62 with Solution
Move Last 3 Characters to Start of String
Write a JavaScript program to move the last three characters to the start of a given string. The string length must be greater than or equal to three.
This JavaScript program takes a string of length at least three and rearranges it by moving the last three characters to the beginning of the string.
Visual Presentation:
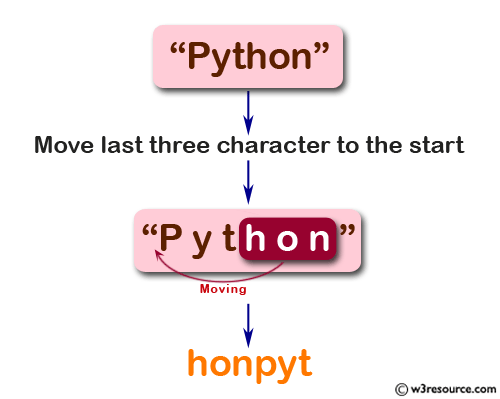
Sample Solution:
JavaScript Code:
// Define a function named right_three with parameter str
function right_three(str) {
// Check if the length of the string is greater than 1
if (str.length > 1) {
// Return the last three characters concatenated with the substring excluding the last three characters
return str.slice(-3) + str.slice(0, -3);
}
// Return the string as is if its length is not greater than 1
return str;
}
// Call the function with sample arguments and log the results to the console
console.log(right_three("Python"));
console.log(right_three("JavaScript"));
console.log(right_three("Hi"));
Output:
honPyt iptJavaScr Hi
Live Demo:
See the Pen JavaScript - Move last three character to the start of a specified string - basic-ex-62 by w3resource (@w3resource) on CodePen.
Flowchart:
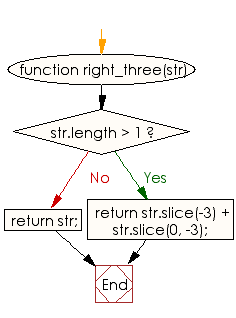
ES6 Version:
// Define a function named right_three with parameter str
const right_three = (str) => {
// Check if the length of str is greater than 1
if (str.length > 1) {
// Use slice to get the last three characters and concatenate with the substring excluding the last three characters
return str.slice(-3) + str.slice(0, -3);
}
// Return str if its length is not greater than 1
return str;
};
// Call the function with sample arguments and log the results to the console
console.log(right_three("Python"));
console.log(right_three("JavaScript"));
console.log(right_three("Hi"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that moves the last three characters of a string to its beginning if the string’s length is at least three.
- Write a JavaScript function that reorders a string by slicing the last three characters and prepending them to the remainder.
- Write a JavaScript program that checks a string's length and then creates a new string by moving its last three characters to the front.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to concatenate two strings except their first character.
Next: JavaScript program to create a string using the middle three characters of a given string of odd length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.