JavaScript: Create a new string without the first and last character of a given string
JavaScript Basic: Exercise-60 with Solution
Remove First and Last Characters in String
Write a JavaScript program to create a new string without the first and last characters of a given string.
This JavaScript program takes an input string and returns a new string with the first and last characters removed. The length of the input string should be at least 2.
Visual Presentation:
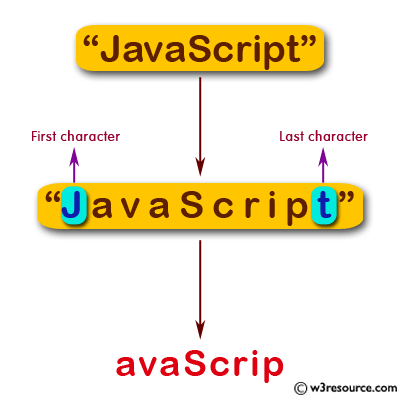
Sample Solution:
JavaScript Code:
Output:
avaScrip H
Live Demo:
Flowchart:
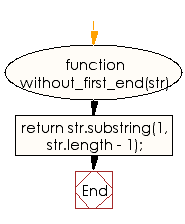
ES6 Version:
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes the first and last characters from a string if its length is greater than 2.
- Write a JavaScript function that uses the slice() method to return a new string without the first and last characters.
- Write a JavaScript program that checks for edge cases before removing the first and last characters from a given string.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to extract the first half of a string of even length.
Next: JavaScript program to concatenate two strings except their first character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.