JavaScript: Check whether a given year is a leap year in the Gregorian calendar
JavaScript Basic: Exercise-6 with Solution
Check Leap Year (Gregorian Calendar)
Write a JavaScript program to determine whether a given year is a leap year in the Gregorian calendar.
The JavaScript program checks if a given year is a leap year by determining if it is divisible by 4 but not by 100, or if it is divisible by 400. It uses conditional statements to implement these rules and then prints whether the year is a leap year or not.
Visual Presentation:
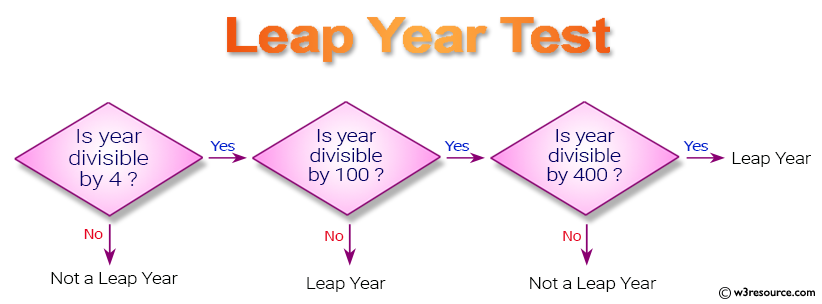
Sample Solution-1:
JavaScript Code:
// Define a function to check if a given year is a leap year
function leapyear(year) {
// Return true if the year is divisible by 4 but not divisible by 100 unless it's also divisible by 400
return (year % 100 === 0) ? (year % 400 === 0) : (year % 4 === 0);
}
// Test the function with various years and log the results to the console
console.log(leapyear(2016)); // Expected output: true
console.log(leapyear(2000)); // Expected output: true
console.log(leapyear(1700)); // Expected output: false
console.log(leapyear(1800)); // Expected output: false
console.log(leapyear(100)); // Expected output: false
Output:
true true false false false
Live Demo:
See the Pen JavaScript: Find Leap Year - basic-ex-6 by w3resource (@w3resource) on CodePen.
Explanation:
A leap year is a year containing one additional day added to keep the calendar year synchronized with the astronomical or seasonal year. Because seasons and astronomical events do not repeat in a whole number of days, calendars that have the same number of days in each year drift over time with respect to the event that the year is supposed to track. By inserting an additional day or month into the year, the drift can be corrected. A year that is not a leap year is called a common year.
Every year that is exactly divisible by four is a leap year, except for years that are exactly divisible by 100, but these centurial years are leap years if they are exactly divisible by 400. For example, the years 1700, 1800, and 1900 are not leap years, but the year 2000 is.
To determine whether a year is a leap year, follow these steps :
Step-1 : If the year is evenly divisible by 4, go to step 2. Otherwise, go to step 5.
Step-2 : If the year is evenly divisible by 100, go to step 3. Otherwise, go to step 4.
Step-3 : If the year is evenly divisible by 400, go to step 4. Otherwise, go to step 5.
Step-4 : The year is a leap year (it has 366 days).
Step-5 : The year is not a leap year (it has 365 days).
Flowchart:
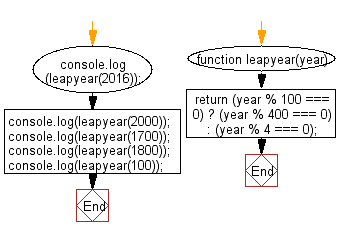
ES6 Version:
// Define a function named leapyear that takes a year as a parameter
const leapyear = (year) => {
// Use the ternary operator to check if the year is a leap year
return (year % 100 === 0) ? (year % 400 === 0) : (year % 4 === 0);
};
// Test the function with sample years
console.log(leapyear(2016));
console.log(leapyear(2000));
console.log(leapyear(1700));
console.log(leapyear(1800));
console.log(leapyear(100));
Sample Solution-2:
- Use new Date(), setting the date to February 29th of the given year.
- Use Date.prototype.getMonth() to check if the month is equal to 1.
JavaScript Code:
// Define an arrow function to check if a given year is a leap year
const is_leapyear = year => new Date(year, 1, 29).getMonth() === 1;
// Test the function with various years and log the results to the console
console.log(is_leapyear(2016)); // Expected output: true
console.log(is_leapyear(2000)); // Expected output: true
console.log(is_leapyear(1700)); // Expected output: false
console.log(is_leapyear(1800)); // Expected output: false
console.log(is_leapyear(100)); // Expected output: false
Output:
true true false false false
Improve this sample solution and post your code through Disqus.
Previous: Rotate the string 'w3resource' in right direction by periodically removing one letter from the end of the string and attaching it to the front.
Next: JavaScript program to find which 1st January is being a Sunday between 2014 and 2050.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics