JavaScript: Extract the first half of a string of even length
JavaScript Basic: Exercise-59 with Solution
Write a JavaScript program to extract the first half of a even string.
This JavaScript program takes an input string with an even number of characters and returns a new string containing only the first half of the original string.
Visual Presentation:
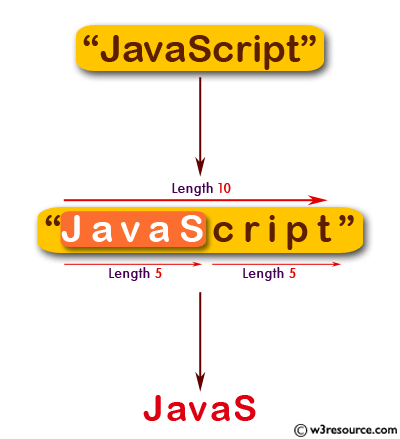
Sample Solution:
JavaScript Code:
// Define a function named first_half with parameter str
function first_half(str) {
// Check if the length of the string is even
if (str.length % 2 == 0) {
// Use the slice method to get the first half of the string
return str.slice(0, str.length / 2);
}
// If the length is odd, return the original string
return str;
}
// Call the function with sample arguments and log the results to the console
console.log(first_half("Python")); // Outputs "Py"
console.log(first_half("JavaScript")); // Outputs "Java"
console.log(first_half("PHP")); // Outputs "PHP"
Output:
Pyt JavaS PHP
Live Demo:
See the Pen JavaScript - Extract the first half of a string of even length - basic-ex-59 by w3resource (@w3resource) on CodePen.
Flowchart:
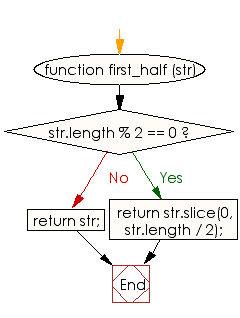
ES6 Version:
// Define a function named first_half with parameter str
const first_half = (str) => {
// Check if the length of the string is even
if (str.length % 2 === 0) {
// Use slice to get the first half of the string
return str.slice(0, str.length / 2);
}
// Return the original string if the length is odd
return str;
};
// Call the function with sample arguments and log the results to the console
console.log(first_half("Python"));
console.log(first_half("JavaScript"));
console.log(first_half("PHP"));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create a new string of 4 copies of the last 3 characters of a given original string.
Next: JavaScript program to create a new string without the first and last character of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-basic-exercise-59.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics