JavaScript: Create a new string of 4 copies of the last 3 characters of a given original string
JavaScript Basic: Exercise-58 with Solution
Four Copies of Last 3 Characters
Write a JavaScript program to create an updated string of 4 copies of the last 3 characters of a given string. The string length must be 3 and above.
This JavaScript program generates a new string by taking the last 3 characters of a given string (if its length is at least 3) and repeating these characters 4 times, creating a string of 12 characters in total.
Visual Presentation:
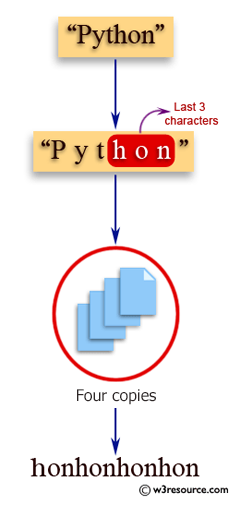
Sample Solution:
JavaScript Code:
// Define a function named newstring with parameter str
function newstring(str) {
// Check if the length of str is greater than or equal to 3
if (str.length >= 3) {
// Extract the last 3 characters of str
result_str = str.substring(str.length - 3);
// Return a new string containing the last 3 characters repeated four times
return result_str + result_str + result_str + result_str;
} else {
// Return false if the length of str is less than 3
return false;
}
}
// Call the function with sample arguments and log the results to the console
console.log(newstring("Python 3.0"));
console.log(newstring("JS"));
console.log(newstring("JavaScript"));
Output:
3.03.03.03.0 false iptiptiptipt
Live Demo:
See the Pen JavaScript - Create a new string of 4 copies of the last 3 characters of a string - basic-ex-57 by w3resource (@w3resource) on CodePen.
Flowchart:
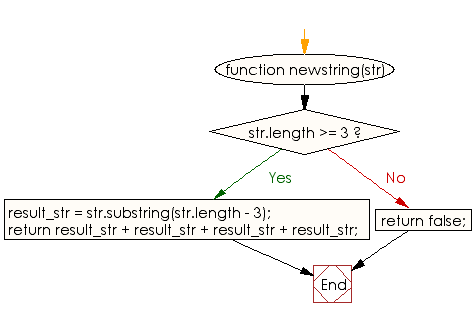
ES6 Version:
// Define a function named newstring with parameter str
const newstring = (str) => {
// Check if the length of the string is greater than or equal to 3
if (str.length >= 3) {
// Use template literals to repeat the last three characters of the string
return `${str.slice(-3)}${str.slice(-3)}${str.slice(-3)}${str.slice(-3)}`;
} else {
// Return false if the length is less than 3
return false;
}
};
// Call the function with sample arguments and log the results to the console
console.log(newstring("Python 3.0"));
console.log(newstring("JS"));
console.log(newstring("JavaScript"));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create a new string of specified copies (positive number) of a given string.
Next: JavaScript program to extract the first half of a string of even length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics