JavaScript: Divide two positive numbers and return a string with properly formatted commas
JavaScript Basic: Exercise-56 with Solution
Divide Numbers and Format with Commas
Write a JavaScript program to divide two positive numbers and return the result as string with properly formatted commas.
This JavaScript program divides two positive numbers and formats the result with commas for thousands, millions, etc. It first performs the division and then converts the result to a string with commas added at appropriate places for better readability.
Sample Solution:
JavaScript Code:
// Function to divide two numbers and format the result with commas
function division_string_format(number1, number2) {
// Check if the divisor is zero
if (number2 === 0) {
return "Cannot divide by zero";
}
// Perform division
let result = number1 / number2;
// Format result with commas
let formattedResult = result.toLocaleString(undefined, {maximumFractionDigits: 2});
// Return the formatted result
return formattedResult;
}
// Example usage:
// Define the dividend and divisor
let dividend = 1000000;
let divisor = 107;
// Perform division and format the result
let result = division_string_format(dividend, divisor);
// Print the result
console.log("Result: " + result);
Output:
Result: 9,345.79
Explanation:
In the exercise above,
- The "division_string_format()" function takes two parameters, 'number1' and 'number2', representing the dividend and divisor respectively.
- It checks if the divisor is zero and returns an error message if so.
- It then performs division and stores the result in the 'result' variable.
- The "toLocaleString()" method formats the result with commas.
- The formatted result is returned as a string.
Live Demo:
See the Pen JavaScript - Divide two positive numbers and return a string with formatted commas - basic-ex-56 by w3resource (@w3resource) on CodePen.
.Flowchart:
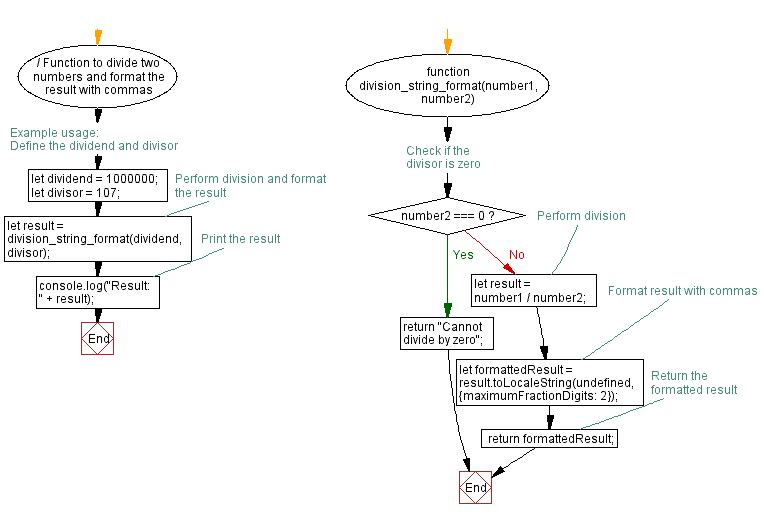
For more Practice: Solve these Related Problems:
- Write a JavaScript program that divides two positive numbers and returns the result as a string with commas correctly placed as thousand separators.
- Write a JavaScript function that performs division and formats both integer and decimal parts with commas where appropriate.
- Write a JavaScript program that handles large numbers by dividing them and then converting the quotient into a human-readable comma-separated format.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check if a given string contains equal number of p's and t's present.
Next: JavaScript program to create a new string of specified copies (positive number) of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.