JavaScript: Check whether a given string contains equal number of p's and t's
JavaScript Basic: Exercise-55 with Solution
Check Equal Number of 'p's and 't's
Write a JavaScript program to check whether a given string contains an equal number of p's and t's.
This JavaScript program checks if a given string has an equal number of 'p' and 't' characters. It counts the occurrences of each character in the string and then compares the two counts to determine if they are equal.
Visual Presentation:
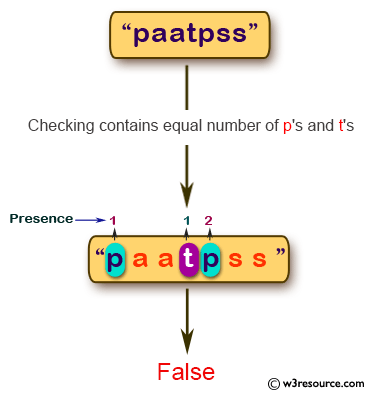
Sample Solution:
JavaScript Code:
// Define a function named equal_pt with parameter str
function equal_pt(str) {
// Replace all characters except 'p' with an empty string and store the result in str_p
var str_p = str.replace(/[^p]/g, "");
// Replace all characters except 't' with an empty string and store the result in str_t
var str_t = str.replace(/[^t]/g, "");
// Get the length of str_p and store it in p_num
var p_num = str_p.length;
// Get the length of str_t and store it in s_num
var s_num = str_t.length;
// Return true if the lengths of str_p and str_t are equal; otherwise, return false
return p_num === s_num;
}
// Log the result of calling equal_pt with the given strings to the console
console.log(equal_pt("paatpss"));
console.log(equal_pt("paatps"));
Output:
false false
Live Demo:
See the Pen JavaScript - Check whether a given string contains equal number of p's and t's - basic-ex-55 by w3resource (@w3resource) on CodePen.
Flowchart:
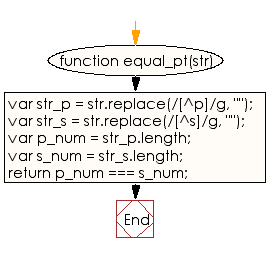
ES6 Version:
// Define a function named equal_pt with parameter str
const equal_pt = (str) => {
// Use regular expressions to replace characters other than 'p' with an empty string
const str_p = str.replace(/[^p]/g, "");
// Use regular expressions to replace characters other than 't' with an empty string
const str_t = str.replace(/[^t]/g, "");
// Get the lengths of the modified strings
const p_num = str_p.length;
const s_num = str_t.length;
// Return whether the lengths of the 'p' and 't' strings are equal
return p_num === s_num;
};
// Log the result of calling equal_pt with the given strings to the console
console.log(equal_pt("paatpss"));
console.log(equal_pt("paatps"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that verifies whether the number of occurrences of the letters 'p' and 't' in a string are equal, ignoring case sensitivity.
- Write a JavaScript function that removes all non-alphabet characters from a string before comparing the counts of 'p' and 't'.
- Write a JavaScript program that takes two characters as input and checks if their frequencies in a given string are identical.
Go to:
PREV : Count Vowels in String.
NEXT : Divide Numbers and Format with Commas.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.