JavaScript: Count the number of vowels in a given string
JavaScript Basic: Exercise-54 with Solution
Write a JavaScript program to count the number of vowels in a given string.
This JavaScript program counts the number of vowels (a, e, i, o, u) in a given string. It iterates through the string, checks each character to see if it is a vowel, and keeps a running total of the vowels encountered.
Visual Presentation:
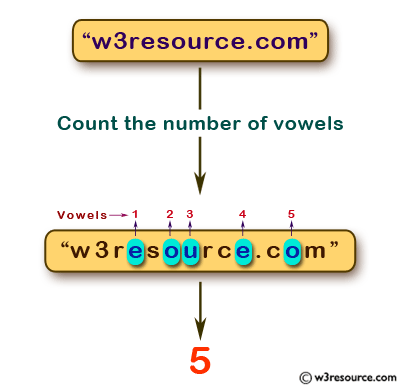
Sample Solution:
JavaScript Code:
// Define a function named vowel_Count with parameter str
function vowel_Count(str) {
// Use regular expression to replace all characters not in 'aeiou' with an empty string
// and get the length of the resulting string, which is the count of vowels
return str.replace(/[^aeiou]/g, "").length;
}
// Log the result of calling vowel_Count with the given strings to the console
console.log(vowel_Count("Python"));
console.log(vowel_Count("w3resource.com"));
Output:
1 5
Live Demo:
See the Pen JavaScript - count the number of vowels in a given string - basic-ex-54 by w3resource (@w3resource) on CodePen.
Flowchart:
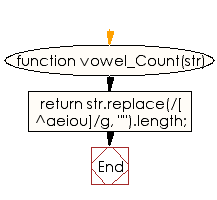
ES6 Version:
// Define a function named vowel_Count with parameter str
const vowel_Count = (str) => {
// Use regular expression to replace non-vowel characters with an empty string,
// and then return the length of the resulting string
return str.replace(/[^aeiou]/g, "").length;
};
// Log the result of calling vowel_Count with the given strings to the console
console.log(vowel_Count("Python"));
console.log(vowel_Count("w3resource.com"));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check if the characters a and b are separated by exactly 3 places anywhere (at least once) in a given string.
Next: JavaScript program to check if a given string contains equal number of p's and t's present.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-basic-exercise-54.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics