JavaScript: Check whether the characters a and b are separated by exactly 3 places anywhere in a given string
JavaScript Basic: Exercise-53 with Solution
Check 'a' and 'b' Separated by Exactly 3 Places
Write a JavaScript program to check whether the characters a and b are separated by exactly 3 places anywhere (at least once) in a given string.
This JavaScript program scans a given string to determine if the characters 'a' and 'b' are separated by exactly three characters. It iterates through the string, checking the positions of 'a' and 'b' to ensure they meet the specified separation condition.
Visual Presentation:
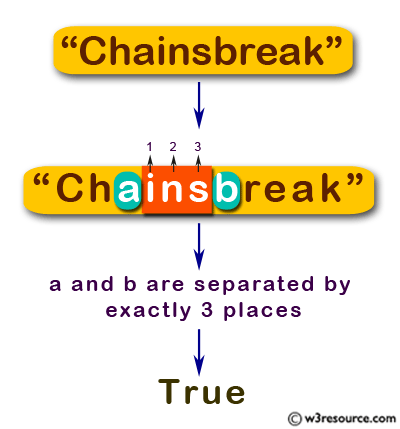
Sample Solution:
JavaScript Code:
// Define a function named ab_Check with parameter str
function ab_Check(str) {
// Use regular expressions to check if the pattern 'a...b' or 'b...a' exists in the given string
// The test() method returns true if the pattern is found, otherwise, it returns false
return (/a...b/).test(str) || (/b...a/).test(str);
}
// Log the result of calling ab_Check with the given strings to the console
console.log(ab_Check("Chainsbreak"));
console.log(ab_Check("pane borrowed"));
console.log(ab_Check("abCheck"));
Output:
true true false
Live Demo:
See the Pen JavaScript - Check whether the characters a and b are separated by exactly 3 places - basic-ex-53 by w3resource (@w3resource) on CodePen.
Flowchart:
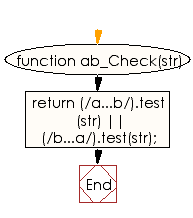
ES6 Version:
// Define a function named ab_Check with parameter str
const ab_Check = (str) => {
// Use regular expressions to test if the string contains either "a...b" or "b...a" patterns
return (/a...b/).test(str) || (/b...a/).test(str);
};
// Log the result of calling ab_Check with the given strings to the console
console.log(ab_Check("Chainsbreak"));
console.log(ab_Check("pane borrowed"));
console.log(ab_Check("abCheck"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if any occurrence of the character 'a' is exactly three positions away from a 'b' in the string, regardless of order.
- Write a JavaScript function that finds all pairs of 'a' and 'b' separated by exactly three characters and returns their starting indices.
- Write a JavaScript program that validates whether a given string contains at least one instance where 'a' and 'b' are exactly three characters apart, ignoring case.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to convert the letters of a given string in alphabetical order.
Next: JavaScript program to count the number of vowels of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.