JavaScript: Convert the letters of a given string in alphabetical order
JavaScript Basic: Exercise-52 with Solution
Sort Letters Alphabetically in String
Write a JavaScript program to convert letters of a given string alphabetically.
This JavaScript program takes a string and sorts its letters in alphabetical order. It splits the string into an array of characters, sorts the array, and then joins the sorted characters back into a string.
Visual Presentation:
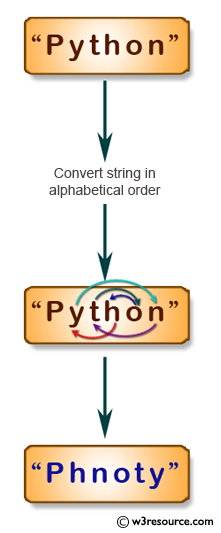
Sample Solution:
JavaScript Code:
// Define a function named alphabet_Soup with parameter str
function alphabet_Soup(str) {
// Split the string into an array of characters, sort the array, and join it back into a string
return str.split("").sort().join("");
}
// Log the result of calling alphabet_Soup with the given strings to the console
console.log(alphabet_Soup("Python"));
console.log(alphabet_Soup("Exercises"));
Output:
Phnoty Eceeirssx
Live Demo:
See the Pen JavaScript - string in alphabetical order - basic-ex-52 by w3resource (@w3resource) on CodePen.
Flowchart:
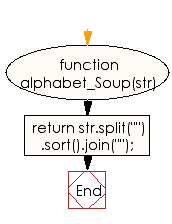
ES6 Version:
// Define a function named alphabet_Soup with parameter str
const alphabet_Soup = (str) => {
// Split the string into an array of characters, sort the array, and join the characters back into a string
return str.split("").sort().join("");
};
// Log the result of calling alphabet_Soup with the given strings to the console
console.log(alphabet_Soup("Python"));
console.log(alphabet_Soup("Exercises"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that sorts only the alphabetic characters of a string while preserving the positions of non-alphabet characters.
- Write a JavaScript function that alphabetically sorts the letters of a string in a case-insensitive manner, then restores the original letter casing.
- Write a JavaScript program that first sorts a string’s letters in alphabetical order and then returns the result in reverse order.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to convert a given number to hours and minutes.
Next: JavaScript program to check if the characters a and b are separated by exactly 3 places anywhere (at least once) in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.