JavaScript: Reverse a given string
JavaScript Basic: Exercise-48 with Solution
Reverse a Given String
Write a JavaScript program to reverse a given string.
This JavaScript program reverses a given string. It iterates through the characters of the string from the last to the first and constructs a new string by appending each character in reverse order. Finally, it returns the reversed string.
Visual Presentation:
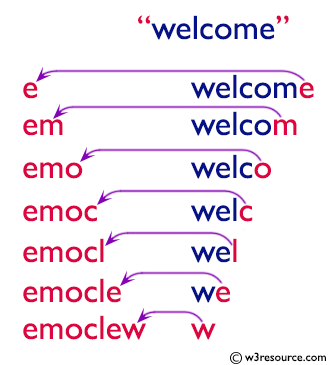
Sample Solution:
JavaScript Code:
// Define a function named string_reverse with a parameter str
function string_reverse(str) {
// Split the string into an array of characters, reverse the order, and join them back into a string
return str.split("").reverse().join("");
}
// Log the result of calling string_reverse with the argument "w3resource" to the console
console.log(string_reverse("w3resource"));
// Log the result of calling string_reverse with the argument "www" to the console
console.log(string_reverse("www"));
// Log the result of calling string_reverse with the argument "JavaScript" to the console
console.log(string_reverse("JavaScript"));
Output:
ecruoser3w www tpircSavaJ
Live Demo:
See the Pen JavaScript - Reverse a string - basic-ex-48 by w3resource (@w3resource) on CodePen.
Flowchart:
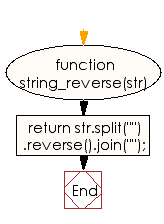
ES6 Version:
// Convert the provided JavaScript code to ES6
// Define a function named string_reverse with parameter str
const string_reverse = (str) => {
// Use split, reverse, and join methods to reverse the characters of the string
return str.split("").reverse().join("");
};
// Log the result of calling string_reverse with the argument "w3resource" to the console
console.log(string_reverse("w3resource"));
// Log the result of calling string_reverse with the argument "www" to the console
console.log(string_reverse("www"));
// Log the result of calling string_reverse with the argument "JavaScript" to the console
console.log(string_reverse("JavaScript"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that reverses a given string without using the built-in reverse() method.
- Write a JavaScript program that reverses each word in a sentence while preserving the word order.
- Write a JavaScript program that reverses a string and then returns the string with alternating character cases.
Go to:
PREV : Check if Integer is in Range 40–10,000.
NEXT : Replace Each Character with Next Alphabet Letter.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.