JavaScript: Check whether a given number presents in the range 40..10000
JavaScript Basic: Exercise-47 with Solution
Check if Integer is in Range 40–10,000
Write a JavaScript program to check whether a given number exists in the range 40..10000.
For example 40 presents in 40 and 4000.
This JavaScript program verifies whether a given number falls within the inclusive range of 40 to 10,000. It checks if the number is greater than or equal to 40 and less than or equal to 10,000. If the number satisfies this condition, it returns true; otherwise, it returns false.
Sample Solution:
JavaScript Code:
// Define a function named test_digit with parameter n
function test_digit(n) {
// Check if n is less than 40 or greater than 10000
if (n < 40 || n > 10000)
// Return false if n is outside the specified range
return false;
else
// Check if n is between 40 and 10000 (inclusive)
if (n >= 40 && n <= 10000)
// Return true if n is within the specified range
return true;
}
// Log the result of calling test_digit with the arguments 40 to the console
console.log(test_digit(45));
// Log the result of calling test_digit with the arguments 79 to the console
console.log(test_digit(79));
// Log the result of calling test_digit with the arguments 30 to the console
console.log(test_digit(30));
Output:
true true false
Explanation:
In the exercise above,
- Function test_digit(n) { ... }: This defines a function named "test_digit()" that takes one parameter 'n'.
- if (n < 40 || n > 10000) return false;: This line checks if the parameter 'n' is less than 40 or greater than 10000. If 'n' is outside this range, the function immediately returns 'false'.
- else if (n >= 40 && n <= 10000) return true;: If the condition in the previous "if" statement is not met (i.e., 'n' is within the range), this line checks if 'n' is between 40 and 10000 (inclusive). If it is, the function returns 'true'.
- console.log(test_digit(45));: This line calls the "test_digit()" function with the argument '45' and logs the result ('true' in this case) to the console.
- console.log(test_digit(79));: Similarly, this line calls the "test_digit()" function with the argument '79' and logs the result ('true' in this case) to the console.
- console.log(test_digit(30));: Finally, this line calls the "test_digit()" function with the argument '30' and logs the result ('false' in this case) to the console.
Live Demo:
See the Pen JavaScript: Check if a number in the range 40..10000 presents in two number - basic-ex-47 by w3resource (@w3resource) on CodePen.
Flowchart:
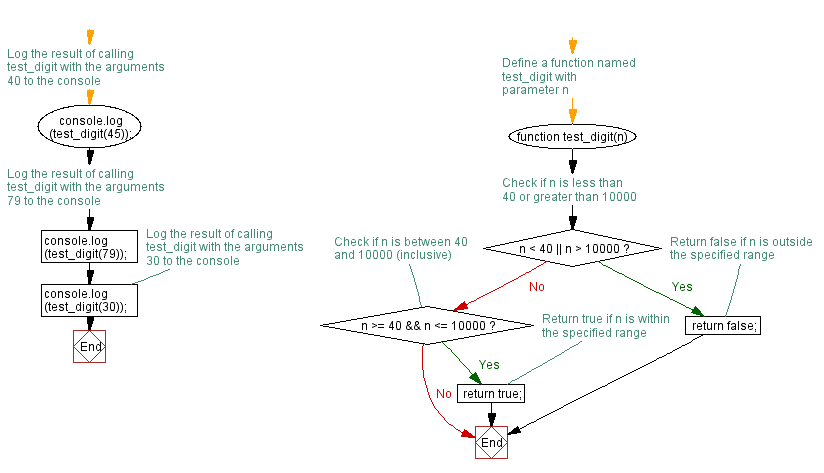
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check two given non-negative integers and if one of the number (not both) is multiple of 7 or 11.
Next: JavaScript program to reverse a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics