JavaScript: Check from three given integers that whether a number is greater than or equal to 20 and less than the other two
JavaScript Basic: Exercise-44 with Solution
Evaluate if Integer is ≥20 and Less Than Another
Write a JavaScript program that evaluates three given integers to determine if any one of them is greater than or equal to 20 and less than the other two.
This JavaScript program evaluates three given integers to determine if one number is greater than or equal to 20 and less than the other two. It compares each number against 20 and ensures that it is not greater than the other two numbers. If these conditions are met for any number, it returns true; otherwise, it returns false.
Visual Presentation:
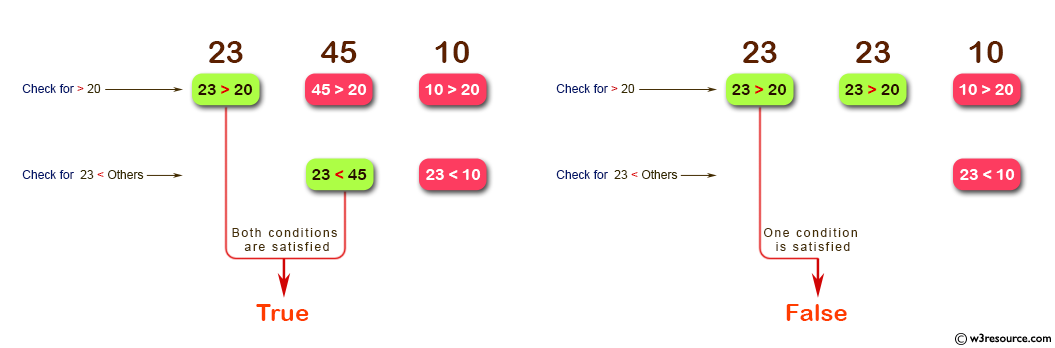
Sample Solution:
JavaScript Code:
// Define a function named lessby20_others with parameters x, y, and z
function lessby20_others(x, y, z) {
// Check if x is greater than or equal to 20 and (x is less than y or x is less than z)
// Check if y is greater than or equal to 20 and (y is less than x or y is less than z)
// Check if z is greater than or equal to 20 and (z is less than y or z is less than x)
return (
(x >= 20 && (x < y || x < z)) ||
(y >= 20 && (y < x || y < z)) ||
(z >= 20 && (z < y || z < x))
);
}
// Log the result of calling lessby20_others with the arguments 23, 45, and 10 to the console
console.log(lessby20_others(23, 45, 10));
// Log the result of calling lessby20_others with the arguments 23, 23, and 10 to the console
console.log(lessby20_others(23, 23, 10));
// Log the result of calling lessby20_others with the arguments 21, 66, and 75 to the console
console.log(lessby20_others(21, 66, 75));
Output:
true false true
Live Demo:
See the Pen JavaScript: Check from three given integers that whether a number is greater than or equal to 20 and less than one of the others - basic-ex-44 by w3resource (@w3resource) on CodePen.
Flowchart:
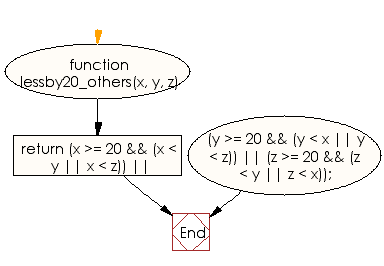
ES6 Version:
// Define a function named lessby20_others using arrow function syntax with parameters x, y, and z
const lessby20_others = (x, y, z) => {
// Return true if x is greater than or equal to 20 and (x is less than y or x is less than z)
// Return true if y is greater than or equal to 20 and (y is less than x or y is less than z)
// Return true if z is greater than or equal to 20 and (z is less than y or z is less than x)
return (x >= 20 && (x < y || x < z)) ||
(y >= 20 && (y < x || y < z)) ||
(z >= 20 && (z < y || z < x));
};
// Log the result of calling lessby20_others with the arguments 23, 45, and 10 to the console
console.log(lessby20_others(23, 45, 10));
// Log the result of calling lessby20_others with the arguments 23, 23, and 10 to the console
console.log(lessby20_others(23, 23, 10));
// Log the result of calling lessby20_others with the arguments 21, 66, and 75 to the console
console.log(lessby20_others(21, 66, 75));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if any one of three integers is exactly 20 and also less than one of the other integers.
- Write a JavaScript program that evaluates three numbers and returns true if one is exactly 20 or if one number is between 20 and the others.
- Write a JavaScript program that checks if at least one of three integers is 20 and is smaller than at least one of the other two.
Go to:
PREV : Check Rightmost Digits of Three Numbers.
NEXT : Check if Integer is 15, or Sum/Difference is 15.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.