JavaScript: Check three given numbers, if the three numbers are same return 30 otherwise return 20 and if two numbers are same return 40
JavaScript Basic: Exercise-41 with Solution
Return 30, 40, or 20 Based on Same Numbers
Write a JavaScript program to check a set of three numbers; if the three numbers are the same return 30; otherwise return 20; and if two numbers are the same return 40.
This JavaScript program checks a set of three numbers. If all three numbers are the same, it returns 30. If two numbers are the same, it returns 40. Otherwise, it returns 20.
Visual Presentation:
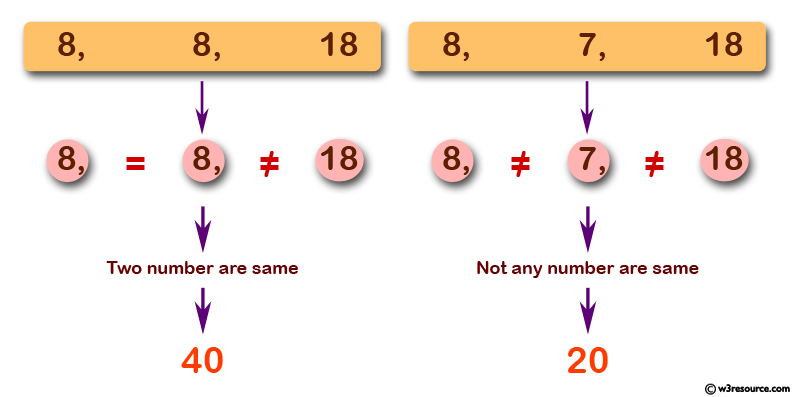
Sample Solution:
JavaScript Code:
// Define a function named three_numbers using function declaration with parameters x, y, and z
function three_numbers(x, y, z) {
// Check if x, y, and z are equal
if (x == y && y == z) {
return 30;
}
// Check if at least two of x, y, and z are equal
if (x == y || y == z || z == x) {
return 40;
}
// Return 20 if none of the conditions are met
return 20;
}
// Log the result of calling three_numbers with the arguments 8, 8, and 8 to the console
console.log(three_numbers(8, 8, 8));
// Log the result of calling three_numbers with the arguments 8, 8, and 18 to the console
console.log(three_numbers(8, 8, 18));
// Log the result of calling three_numbers with the arguments 8, 7, and 18 to the console
console.log(three_numbers(8, 7, 18));
Output:
30 40 20
Live Demo:
See the Pen JavaScript: Check three given numbers - basic-ex-41 by w3resource (@w3resource) on CodePen.
Flowchart:
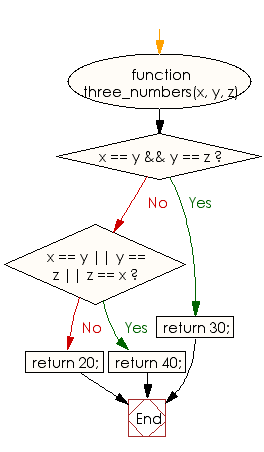
ES6 Version:
// Define a function named three_numbers using arrow function syntax with parameters x, y, and z
const three_numbers = (x, y, z) => {
// Check if x, y, and z are equal
if (x == y && y == z) {
return 30;
}
// Check if at least two of x, y, and z are equal
if (x == y || y == z || z == x) {
return 40;
}
// Return 20 if none of the conditions are met
return 20;
};
// Log the result of calling three_numbers with the arguments 8, 8, and 8 to the console
console.log(three_numbers(8, 8, 8));
// Log the result of calling three_numbers with the arguments 8, 8, and 18 to the console
console.log(three_numbers(8, 8, 18));
// Log the result of calling three_numbers with the arguments 8, 7, and 18 to the console
console.log(three_numbers(8, 7, 18));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that accepts three numbers and returns 30 if all are equal, 40 if exactly two are equal, and 20 if all are distinct.
- Write a JavaScript program that computes a result based on three integers: return 30 if they are identical, 40 if only two match, otherwise 20.
- Write a JavaScript program that evaluates three numbers and returns a unique value based on whether one, two, or all three numbers are the same.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check from two given integers if either one is 8 or their sum or difference is 8.
Next: JavaScript program to check if three given numbers are increasing in strict mode or in soft mode.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.