JavaScript: Compute the sum of the two given integers
JavaScript Basic: Exercise-39 with Solution
Sum Two Integers and Return Based on Range
Write a JavaScript program to compute the sum of the two given integers. If the sum is in the range 50..80 return 65 otherwise return 80.
This JavaScript program calculates the sum of two given integers. If the sum falls within the range of 50 to 80 (inclusive), it returns 65; otherwise, it returns 80.
Visual Presentation:
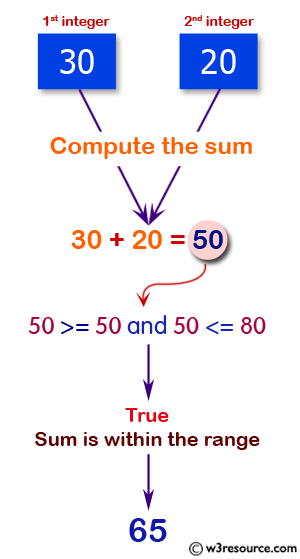
Sample Solution:
JavaScript Code:
// Define a function named sortaSum with parameters x and y
function sortaSum(x, y)
{
// Calculate the sum of x and y and store it in the variable sum_nums
const sum_nums = x + y;
// Check if the sum_nums is between 50 and 80 (inclusive)
if (sum_nums >= 50 && sum_nums <= 80) {
// If true, return 65
return 65;
}
// If the condition is not met, return 80
return 80;
}
// Log the result of calling sortaSum with the arguments 30 and 20 to the console
console.log(sortaSum(30, 20));
// Log the result of calling sortaSum with the arguments 90 and 80 to the console
console.log(sortaSum(90, 80));
Output:
65 80
Live Demo:
See the Pen JavaScript: compute the sum of the two given integers - basic-ex-39 by w3resource (@w3resource) on CodePen.
Flowchart:
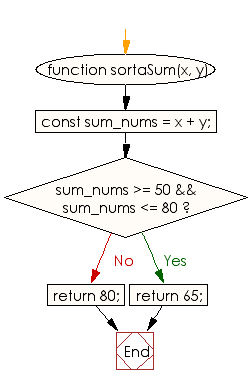
ES6 Version:
// Define a function named sortaSum using arrow function syntax with parameters x and y
const sortaSum = (x, y) => {
// Calculate the sum of x and y
const sum_nums = x + y;
// Check if the sum is between 50 and 80 (inclusive)
if (sum_nums >= 50 && sum_nums <= 80) {
// Return 65 if the condition is true
return 65;
}
// Return 80 if the condition is false
return 80;
};
// Log the result of calling sortaSum with the arguments 30 and 20 to the console
console.log(sortaSum(30, 20));
// Log the result of calling sortaSum with the arguments 90 and 80 to the console
console.log(sortaSum(90, 80));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that sums two integers and returns a specific value based on whether the sum falls within a custom range.
- Write a JavaScript program that computes the sum of two numbers and conditionally returns 65 or 80 based on a dynamic range check.
- Write a JavaScript program that calculates the sum of two integers and uses a ternary operator to return one of two values depending on the sum's range.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check the total marks of a student in various examinations.Next: JavaScript program to check from two given integers if either one is 8 or their sum or difference is 8.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.