JavaScript: Create new string with first 3 characters are in lower case
JavaScript Basic: Exercise-37 with Solution
Modify String Based on Length (First 3 Lowercase/Uppercase)
Write a JavaScript program to produce a new string that has the first 3 characters in lower case from a given string. If the string length is less than 3 convert all the characters to upper case.
The program converts the first three characters of a given string to lowercase if the string length is 3 or more. If the string length is less than 3, it converts the entire string to uppercase.
Visual Presentation:
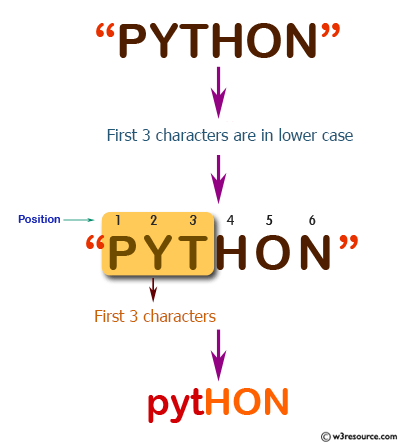
Sample Solution:
JavaScript Code:
// Define a function named upper_lower with parameter str
function upper_lower(str) {
// Check if the length of str is less than 3
if (str.length < 3) {
// If true, return the uppercase version of the entire string
return str.toUpperCase();
}
// Create a variable named front_part and store the lowercase version of the first 3 characters of str
front_part = (str.substring(0, 3)).toLowerCase();
// Create a variable named back_part and store the substring of str from index 3 to the end
back_part = str.substring(3, str.length);
// Return the concatenation of front_part and back_part
return front_part + back_part;
}
// Log the result of calling upper_lower with the argument "Python" to the console
console.log(upper_lower("Python"));
// Log the result of calling upper_lower with the argument "Py" to the console
console.log(upper_lower("Py"));
// Log the result of calling upper_lower with the argument "JAVAScript" to the console
console.log(upper_lower("JAVAScript"));
Output:
python PY javAScript
Live Demo:
See the Pen JavaScript: check if the last digit of the three given positive integers is same - basic-ex-36 by w3resource (@w3resource) on CodePen.
Flowchart:
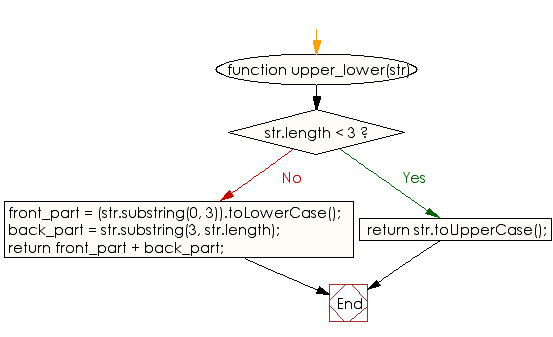
ES6 Version:
// Define a function named upper_lower using arrow function syntax with parameter str
const upper_lower = (str) => {
// Check if the length of str is less than 3
if (str.length < 3) {
// Return str converted to uppercase
return str.toUpperCase();
}
// Create front_part as a lowercase substring of the first 3 characters of str
const front_part = str.substring(0, 3).toLowerCase();
// Create back_part as a substring of str from index 3 to the end
const back_part = str.substring(3);
// Return the concatenation of front_part and back_part
return front_part + back_part;
};
// Log the result of calling upper_lower with the argument "Python" to the console
console.log(upper_lower("Python"));
// Log the result of calling upper_lower with the argument "Py" to the console
console.log(upper_lower("Py"));
// Log the result of calling upper_lower with the argument "JAVAScript" to the console
console.log(upper_lower("JAVAScript"));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check if the last digit of the three given positive integers is same.
Next: JavaScript program to check the total marks of a student in various examinations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics