JavaScript: Check whether the last digit of the three given positive integers is same
JavaScript Basic: Exercise-36 with Solution
Check if Last Digit of Three Integers is Same
Write a JavaScript program that checks whether the last digit of three positive integers is the same.
The program compares the last digits of three given positive integers. It returns true if all three integers share the same last digit, and false otherwise.
Visual Presentation:
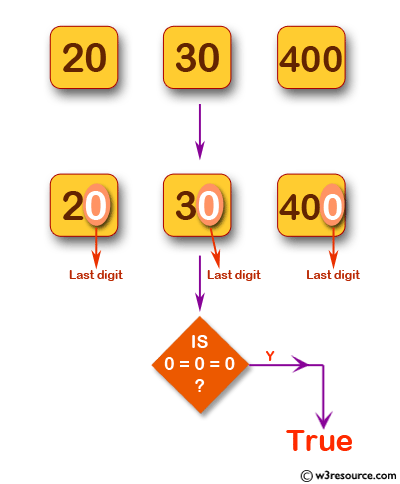
Sample Solution:
JavaScript Code:
// Define a function named last_digit with parameters x, y, and z
function last_digit(x, y, z) {
// Check if x, y, and z are greater than 0
if (x > 0 && y > 0 && z > 0) {
// Check if the last digits of x, y, and z are equal
return x % 10 === y % 10 && y % 10 === z % 10 && x % 10 === z % 10;
} else {
// If any of the numbers is not greater than 0, return false
return false;
}
}
// Log the result of calling last_digit with the arguments 20, 30, and 400 to the console
console.log(last_digit(20, 30, 400));
// Log the result of calling last_digit with the arguments -20, 30, and 400 to the console
console.log(last_digit(-20, 30, 400));
// Log the result of calling last_digit with the arguments 20, -30, and 400 to the console
console.log(last_digit(20, -30, 400));
// Log the result of calling last_digit with the arguments 20, 30, and -400 to the console
console.log(last_digit(20, 30, -400));
Output:
true false false false
Live Demo:
See the Pen JavaScript: check whether the last digit of the three given positive integers is same - basic-ex-36 by w3resource (@w3resource) on CodePen.
Flowchart:
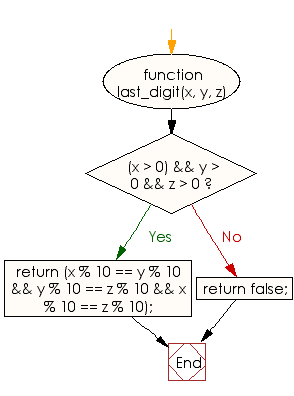
ES6 Version:
// Define a function named last_digit using arrow function syntax with parameters x, y, and z
const last_digit = (x, y, z) => {
// Check if all numbers are positive
if (x > 0 && y > 0 && z > 0) {
// Check if the last digits of x, y, and z are equal
return x % 10 === y % 10 && y % 10 === z % 10 && x % 10 === z % 10;
} else {
// Return false if any number is not positive
return false;
}
};
// Log the result of calling last_digit with the arguments 20, 30, and 400 to the console
console.log(last_digit(20, 30, 400));
// Log the result of calling last_digit with the arguments -20, 30, and 400 to the console
console.log(last_digit(-20, 30, 400));
// Log the result of calling last_digit with the arguments 20, -30, and 400 to the console
console.log(last_digit(20, -30, 400));
// Log the result of calling last_digit with the arguments 20, 30, and -400 to the console
console.log(last_digit(20, 30, -400));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether a given string contains 2 to 4 numbers of a specified character.
Next: JavaScript program to create a new string with first 3 characters are in lower case from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics