JavaScript: Check a given string contains 2 to 4 numbers of a specified character
JavaScript Basic: Exercise-35 with Solution
Check Character Between 2nd and 4th Positions in String
Write a program to check whether a specified character exists between the 2nd and 4th positions in a given string.
The program checks if a specified character is present in a given string between the 2nd and 4th positions (i.e., indices 1 to 3). It returns true if the character exists in that range and false otherwise.
Sample Solution:
JavaScript Code:
// Define a function named check_char with parameters str1 (a string) and char (a character)
function check_char(str1, char)
{
// Initialize a counter variable to 0
let ctr = 0;
// Use a for loop to iterate through each character in the string
for (let i = 0; i < str1.length; i++)
{
// Check if the current character is equal to the specified char and if the index is between 1 and 3 (inclusive)
if ((str1.charAt(i) == char) && (i >= 1 && i <= 3))
{
// Set the counter to 1 and break out of the loop
ctr = 1;
break;
}
}
// Check if the counter is 1 and return true, otherwise return false
if (ctr == 1) return true;
return false;
}
// Log the result of calling check_char with the arguments "Python" and "y" to the console
console.log(check_char("Python", "y"));
// Log the result of calling check_char with the arguments "JavaScript" and "a" to the console
console.log(check_char("JavaScript", "a"));
// Log the result of calling check_char with the arguments "Console" and "o" to the console
console.log(check_char("Console", "o"));
// Log the result of calling check_char with the arguments "Console" and "C" to the console
console.log(check_char("Console", "C"));
// Log the result of calling check_char with the arguments "Console" and "e" to the console
console.log(check_char("Console", "e"));
// Log the result of calling check_char with the arguments "JavaScript" and "S" to the console
console.log(check_char("JavaScript", "S"));
Output:
true true true false false false
Live Demo:
See the Pen JavaScript: check a given string contains 2 to 4 numbers - basic-ex-35 by w3resource (@w3resource) on CodePen.
Flowchart:
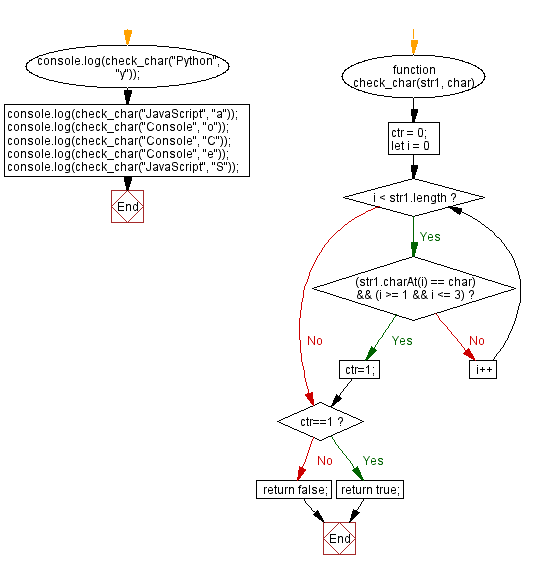
ES6 Version:
// Define a function named check_char using arrow function syntax with parameters str1 and char
const check_char = (str1, char) => {
// Initialize a counter variable to 0
let ctr = 0;
// Use a for loop to iterate through each character in str1
for (let i = 0; i < str1.length; i++) {
// Check if the current character equals char and the index is between 1 and 3 (inclusive)
if (str1.charAt(i) === char && i >= 1 && i <= 3) {
// Set the counter to 1 and break out of the loop
ctr = 1;
break;
}
}
// Check if the counter is 1 and return true, otherwise return false
return ctr === 1;
};
// Log the result of calling check_char with the arguments "Python" and "y" to the console
console.log(check_char("Python", "y"));
// Log the result of calling check_char with the arguments "JavaScript" and "a" to the console
console.log(check_char("JavaScript", "a"));
// Log the result of calling check_char with the arguments "Console" and "o" to the console
console.log(check_char("Console", "o"));
// Log the result of calling check_char with the arguments "Console" and "C" to the console
console.log(check_char("Console", "C"));
// Log the result of calling check_char with the arguments "Console" and "e" to the console
console.log(check_char("Console", "e"));
// Log the result of calling check_char with the arguments "JavaScript" and "S" to the console
console.log(check_char("JavaScript", "S"));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the larger number from the two given positive integers, the two numbers are in the range 40..60 inclusive.
Next: JavaScript program to check if the last digit of the three given positive integers is same.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics