JavaScript: Find the larger number from the two given positive integers
JavaScript Basic: Exercise-34 with Solution
Find Larger Number in Range 40–60
Write a JavaScript program to find the largest number from the two given positive integers. The two numbers are in the range 40..60 inclusive.
The JavaScript program finds the largest number between two given positive integers, ensuring both numbers are within the range of 40 to 60 inclusive. If both numbers are within the range, it returns the larger number; otherwise, it handles invalid input.
Visual Presentation:
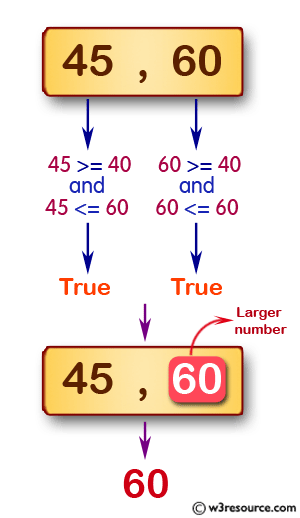
Sample Solution:
JavaScript Code:
// Define a function named max_townums_range with parameters x and y
function max_townums_range(x, y) {
// Check if x and y fall within the specified range using logical AND and comparison operators
if (x >= 40 && x <= 60 && y >= 40 && y <= 60) {
// Check if x and y are the same
if (x === y) {
return "Numbers are the same";
} else if (x > y) {
return x; // Return x if it is greater than y
} else {
return y; // Return y if it is greater than x
}
} else {
return "Numbers don't fit in range"; // Return this message if numbers are outside the range
}
}
// Log the result of calling max_townums_range with the arguments 45 and 60 to the console
console.log(max_townums_range(45, 60));
// Log the result of calling max_townums_range with the arguments 25 and 60 to the console
console.log(max_townums_range(25, 60));
// Log the result of calling max_townums_range with the arguments 45 and 80 to the console
console.log(max_townums_range(45, 80));
Output :
60 Numbers don't fit in range Numbers don't fit in range
Live Demo:
See the Pen javascript-basic-exercise-34 by w3resource (@w3resource) on CodePen.
Flowchart:
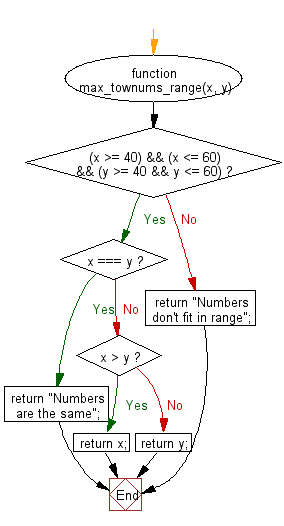
ES6 Version:
// Define a function named max_townums_range using arrow function syntax with parameters x and y
const max_townums_range = (x, y) => {
// Check if x and y fall within the specified ranges using logical AND operators
if (x >= 40 && x <= 60 && y >= 40 && y <= 60) {
// Check if x is equal to y
if (x === y) {
return "Numbers are the same";
} else if (x > y) {
return x;
} else {
return y;
}
} else {
return "Numbers don't fit in range";
}
};
// Log the result of calling max_townums_range with the arguments 45 and 60 to the console
console.log(max_townums_range(45, 60));
// Log the result of calling max_townums_range with the arguments 25 and 60 to the console
console.log(max_townums_range(25, 60));
// Log the result of calling max_townums_range with the arguments 45 and 80 to the console
console.log(max_townums_range(45, 80));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that compares two positive integers within the range 40..60 and returns the larger one.
- Write a JavaScript program that validates if two numbers are within the 40 to 60 range and outputs the greater value.
- Write a JavaScript program that accepts two numbers, checks their range (40-60), and returns the higher number or an error if out of range.
Go to:
PREV : Check if Two Numbers are in Specific Ranges.
NEXT : Check Character Between 2nd and 4th Positions in String.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.