JavaScript: Check whether a string "Script" presents at 5th (index 4) position in a given string
JavaScript Basic: Exercise-30 with Solution
Remove 'Script' from String at 5th Position
Write a JavaScript program to check whether a string "Script" appears at the 5th (index 4) position in a given string. If "Script" appears in the string, return the string without "Script" otherwise return the original one.
This JavaScript program checks if the substring "Script" appears at the 5th position (index 4) in a given string. If "Script" is found at the specified position, it returns the string with "Script" removed; otherwise, it returns the original string.
Visual Presentation:
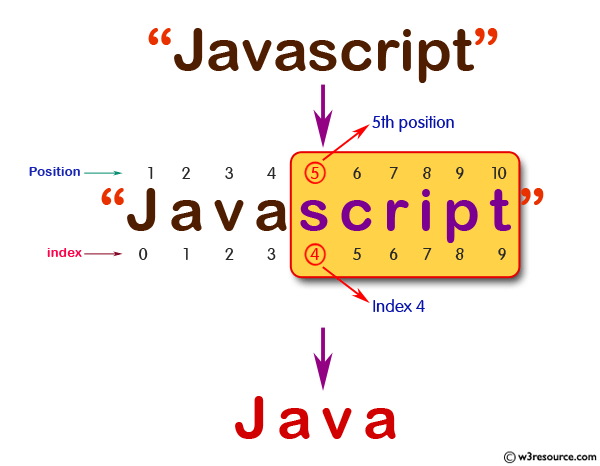
Sample Solution:
JavaScript Code:
// Define a function named check_script that takes a string parameter str
function check_script(str) {
// Check if the length of the string is less than 6
if (str.length < 6) {
// If true, return the original string as it is
return str;
}
// Initialize a variable result_str with the value of the input string
let result_str = str;
// Check if the substring from index 10 to index 4 (exclusive) is equal to 'Script'
if (str.substring(10, 4) == 'Script') {
// If true, update result_str to exclude the substring 'Script' from index 4 to the end
result_str = str.substring(0, 4) + str.substring(10, str.length);
}
// Return the final result_str
return result_str;
}
// Log the result of calling check_script with the argument "JavaScript" to the console
console.log(check_script("JavaScript"));
// Log the result of calling check_script with the argument "CoffeeScript" to the console
console.log(check_script("CoffeeScript"));
Output:
Java CoffeeScript
Live Demo:
See the Pen JavaScript: check if a string Script presents at 5th position - ex-30 by w3resource (@w3resource) on CodePen.
Flowchart:
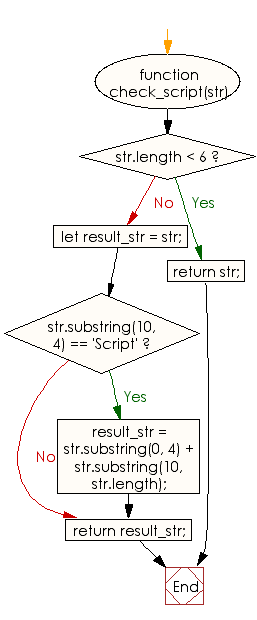
ES6 Version:
// Define a function named check_script that takes a string parameter str
const check_script = (str) => {
// Check if the length of the string is less than 6
if (str.length < 6) {
// If true, return the original string as it is
return str;
}
// Initialize a variable result_str with the value of the input string
let result_str = str;
// Check if the substring from index 10 to index 4 (exclusive) is equal to 'Script'
if (str.substring(10, 4) == 'Script') {
// If true, update result_str to exclude the substring 'Script' from index 4 to the end
result_str = str.substring(0, 4) + str.substring(10, str.length);
}
// Return the final result_str
return result_str;
};
// Log the result of calling check_script with the argument "JavaScript" to the console
console.log(check_script("JavaScript"));
// Log the result of calling check_script with the argument "CoffeeScript" to the console
console.log(check_script("CoffeeScript"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if the substring 'Script' appears starting at the 5th position of a string, and removes it if found.
- Write a JavaScript program that detects 'Script' at any specified index in a string and replaces it with an empty string.
- Write a JavaScript program that searches for 'Script' at the 5th index of a string and removes it, leaving the rest of the string intact.
Go to:
PREV : Check if Three Integers are in Range 50–99.
NEXT : Find Largest of Three Integers.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.