JavaScript : Display the current date
JavaScript Basic : Exercise-3 with Solution
Get Current Date in Various Formats
Write a JavaScript program to get the current date.
Expected Output :
mm-dd-yyyy, mm/dd/yyyy or dd-mm-yyyy, dd/mm/yyyy
This JavaScript program retrieves the current date and formats it in multiple ways (mm-dd-yyyy, mm/dd/yyyy, dd-mm-yyyy, dd/mm/yyyy). It uses the Date object to get the current day, month, and year, and then adds leading zeros if necessary to ensure proper formatting. Finally, it logs the formatted date strings to the console.
Sample Solution:
JavaScript Code:
// Get the current date
var today = new Date();
// Get the day of the month
var dd = today.getDate();
// Get the month (adding 1 because months are zero-based)
var mm = today.getMonth() + 1;
// Get the year
var yyyy = today.getFullYear();
// Add leading zero if the day is less than 10
if (dd < 10) {
dd = '0' + dd;
}
// Add leading zero if the month is less than 10
if (mm < 10) {
mm = '0' + mm;
}
// Format the date as mm-dd-yyyy and log it
today = mm + '-' + dd + '-' + yyyy;
console.log(today);
// Format the date as mm/dd/yyyy and log it
today = mm + '/' + dd + '/' + yyyy;
console.log(today);
// Format the date as dd-mm-yyyy and log it
today = dd + '-' + mm + '-' + yyyy;
console.log(today);
// Format the date as dd/mm/yyyy and log it
today = dd + '/' + mm + '/' + yyyy;
console.log(today);
Output:
01-09-2018 01/09/2018 09-01-2018 09/01/2018
Live Demo:
See the Pen JavaScript program to get the current date - basic-ex-3 by w3resource (@w3resource) on CodePen.
Explanation:
Declaring a JavaScript date : In JavaScript Date objects are based on a time value that is the number of milliseconds since 1 January, 1970 UTC. You can declare a date in the following ways :
new Date(); new Date(value); new Date(dateString); new Date(year, month[, day[, hour[, minutes[, seconds[, milliseconds]]]]]);
The getDate() method is used to get the day of the month for the specified date according to local time. The value returned by getDate() is an integer between 1 and 31.
The getMonth() method returns the month in the specified date according to local time, as a zero-based value (where zero indicates the first month of the year). The value returned by getMonth() is an integer between 0 and 11. 0 corresponds to January, 1 to February, and so on.
The getFullYear() method is used to get the year of the specified date according to local time. The value returned by the method is an absolute number. For dates between the years 1000 and 9999, getFullYear() returns a four-digit number, for example, 1985.
Flowchart:
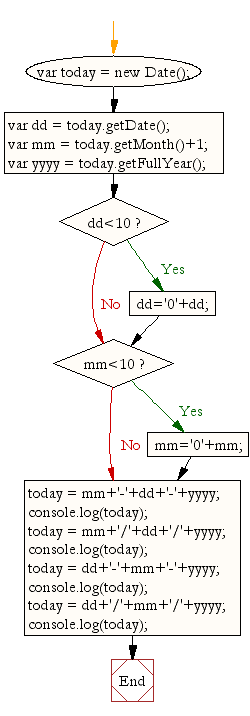
ES6 Version:
let today = new Date();
let dd = today.getDate();
let mm = today.getMonth()+1;
const yyyy = today.getFullYear();
if(dd<10)
{
dd=`0${dd}`;
}
if(mm<10)
{
mm=`0${mm}`;
}
today = `${mm}-${dd}-${yyyy}`;
console.log(today);
today = `${mm}/${dd}/${yyyy}`;
console.log(today);
today = `${dd}-${mm}-${yyyy}`;
console.log(today);
today = `${dd}/${mm}/${yyyy}`;
console.log(today);
Improve this sample solution and post your code through Disqus.
Previous: JavaScript function to print the contents of the current window.
Next: JavaScript function to find the area of a triangle where lengths of the three of its sides are 5, 6, 7.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics