JavaScript: Check whether three given integer values are in the range 50..99
JavaScript Basic: Exercise-29 with Solution
Check if Three Integers are in Range 50–99
Write a JavaScript program to check whether three given integer values are in the range 50..99 (inclusive). Return true if one or more of them are in the specified range.
This JavaScript program checks if any of three given integers fall within the range of 50 to 99 (inclusive). It evaluates each integer to see if it is within this range and returns true if at least one of the integers meets the condition.
Visual Presentation:
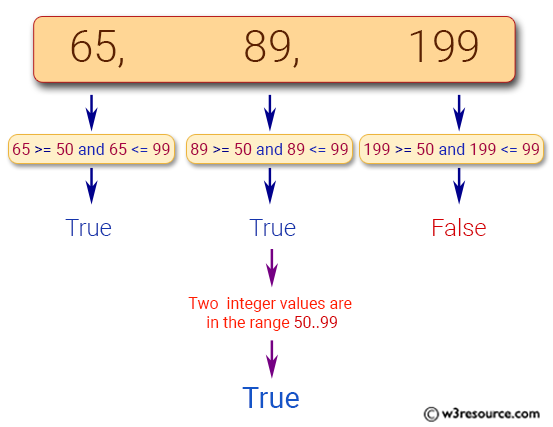
Sample Solution:
JavaScript Code:
// Define a function named check_three_nums that takes three parameters x, y, and z
function check_three_nums(x, y, z) {
// Check if at least one of the numbers is in the range between 50 and 99 (inclusive)
return (x >= 50 && x <= 99) || (y >= 50 && y <= 99) || (z >= 50 && z <= 99);
}
// Log the result of calling the check_three_nums function with the arguments 50, 90, and 99 to the console
console.log(check_three_nums(50, 90, 99));
// Log the result of calling the check_three_nums function with the arguments 5, 9, and 199 to the console
console.log(check_three_nums(5, 9, 199));
// Log the result of calling the check_three_nums function with the arguments 65, 89, and 199 to the console
console.log(check_three_nums(65, 89, 199));
// Log the result of calling the check_three_nums function with the arguments 65, 9, and 199 to the console
console.log(check_three_nums(65, 9, 199));
Output:
true false true true
Live Demo:
See the Pen JavaScript: check if three given integer values are in a range-basic- ex-29 by w3resource (@w3resource) on CodePen.
Flowchart:
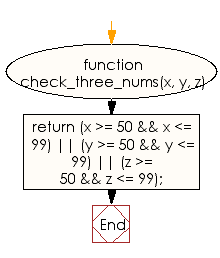
ES6 Version:
// Define a function named check_three_nums using arrow function syntax, which takes three parameters x, y, and z
const check_three_nums = (x, y, z) => (x >= 50 && x <= 99) || (y >= 50 && y <= 99) || (z >= 50 && z <= 99);
// Log the result of calling the check_three_nums function with the arguments 50, 90, and 99 to the console
console.log(check_three_nums(50, 90, 99));
// Log the result of calling the check_three_nums function with the arguments 5, 9, and 199 to the console
console.log(check_three_nums(5, 9, 199));
// Log the result of calling the check_three_nums function with the arguments 65, 89, and 199 to the console
console.log(check_three_nums(65, 89, 199));
// Log the result of calling the check_three_nums function with the arguments 65, 9, and 199 to the console
console.log(check_three_nums(65, 9, 199));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check if two given integer values are in the range 50..99
Next: JavaScript program to check if a string "Script" presents at 5th (index 4) position in a given string,
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics