JavaScript: Check whether a string starts with 'Java' and false otherwise
JavaScript Basic: Exercise-27 with Solution
Check if String Starts with 'Java'
Write a JavaScript program to check whether a string starts with 'Java' if it does not otherwise.
This JavaScript program checks whether a given string starts with 'Java'. It uses a method to determine if the string begins with the specified substring 'Java'. If the string starts with 'Java', it returns true; otherwise, it returns false. This approach efficiently handles the comparison, providing a clear and concise solution.
Visual Presentation:
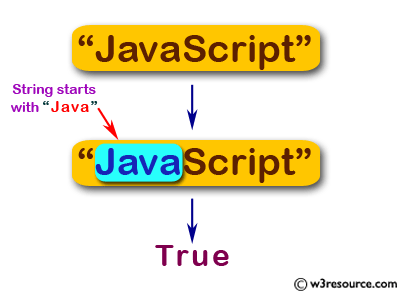
Sample Solution:
JavaScript Code:
// Define a function named start_spec_str that takes a parameter str
function start_spec_str(str) {
// Check if the length of str is less than 4
if (str.length < 4) {
// If true, return false
return false;
}
// Extract the first four characters of str
front = str.substring(0, 4);
// Check if front is equal to 'Java'
if (front == 'Java') {
// If true, return true
return true;
} else {
// If not equal to 'Java', return false
return false;
}
}
// Log the result of calling the start_spec_str function with the argument "JavaScript" to the console
console.log(start_spec_str("JavaScript"));
// Log the result of calling the start_spec_str function with the argument "Java" to the console
console.log(start_spec_str("Java"));
// Log the result of calling the start_spec_str function with the argument "Python" to the console
console.log(start_spec_str("Python"));
Output:
true true false
Live Demo:
See the Pen JavaScript: check if a string starts with Java -basic- ex-27 by w3resource (@w3resource) on CodePen.
Flowchart:
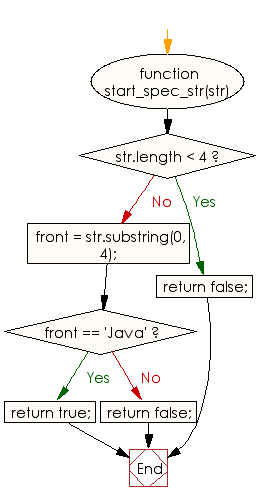
ES6 Version:
// Using ES6 arrow function syntax to define the start_spec_str function
const start_spec_str = (str) => {
// Check if the length of str is less than 4
if (str.length < 4) {
// If true, return false
return false;
}
// Extract the first four characters of str
const front = str.substring(0, 4);
// Check if front is equal to 'Java'
if (front === 'Java') {
// If true, return true
return true;
} else {
// If not equal to 'Java', return false
return false;
}
};
// Log the result of calling the start_spec_str function with the argument "JavaScript" to the console
console.log(start_spec_str("JavaScript"));
// Log the result of calling the start_spec_str function with the argument "Java" to the console
console.log(start_spec_str("Java"));
// Log the result of calling the start_spec_str function with the argument "Python" to the console
console.log(start_spec_str("Python"));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create a new string from a given string taking the last 3 characters and added at both the front and back.
Next: JavaScript program to check if two given integer values are in the range 50..99 .
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics