JavaScript: Create a new string from a given string taking the last 3 characters and added at both the front and back
JavaScript Basic: Exercise-26 with Solution
Add Last 3 Characters to Front and Back of String
Write a JavaScript program to create a string from a given string. This is done by taking the last 3 characters and adding them at both the front and back. The string length must be 3 or more.
This JavaScript program creates a new string from a given string by taking its last three characters and appending them both at the front and back. It first checks if the length of the given string is three or more characters to ensure the operation is valid. Then, it extracts the last three characters and concatenates them with the original string, forming the desired result.
Visual Presentation:
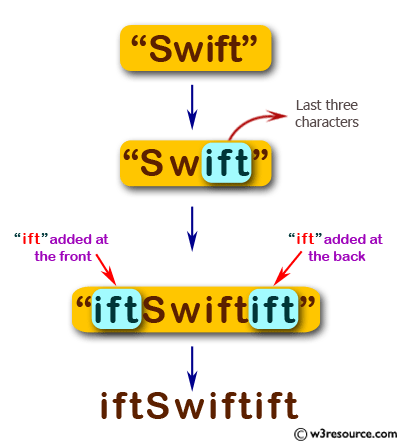
Sample Solution:
JavaScript Code:
// Define a function named front_back3 that takes a parameter str
function front_back3(str) {
// Check if the length of str is greater than or equal to 3
if (str.length >= 3) {
// Set str_len to 3
str_len = 3;
// Extract the last three characters of str
back = str.substring(str.length - 3);
// Return the concatenation of back, str, and back
return back + str + back;
} else {
// If the length of str is less than 3, return false
return false;
}
}
// Log the result of calling the front_back3 function with the argument "abc" to the console
console.log(front_back3("abc"));
// Log the result of calling the front_back3 function with the argument "ab" to the console
console.log(front_back3("ab"));
// Log the result of calling the front_back3 function with the argument "abcd" to the console
console.log(front_back3("abcd"));
Output:
abcabcabc false bcdabcdbcd
Live Demo:
See the Pen JavaScript: added characters added at both the front and back-basic- ex-25 by w3resource (@w3resource) on CodePen.
Flowchart:
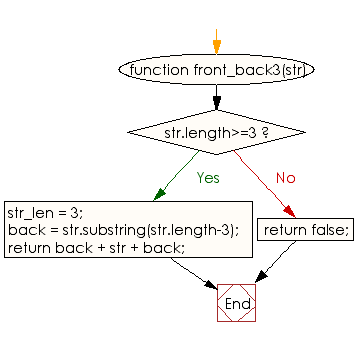
ES6 Version:
// Using ES6 arrow function syntax to define the front_back3 function
const front_back3 = (str) => {
// Check if the length of str is greater than or equal to 3
if (str.length >= 3) {
// Set str_len to 3
const str_len = 3;
// Extract the last three characters of str
const back = str.substring(str.length - 3);
// Return the concatenation of back, str, and back
return back + str + back;
} else {
// If the length of str is less than 3, return false
return false;
}
};
// Log the result of calling the front_back3 function with the argument "abc" to the console
console.log(front_back3("abc"));
// Log the result of calling the front_back3 function with the argument "ab" to the console
console.log(front_back3("ab"));
// Log the result of calling the front_back3 function with the argument "abcd" to the console
console.log(front_back3("abcd"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that takes a string and adds its last three characters to both the beginning and end, ensuring the string length is sufficient.
- Write a JavaScript program that, if the string length is greater than 5, adds the last three characters to the front and back; otherwise, adds the entire string.
- Write a JavaScript program that concatenates the last three characters of a string to both ends and then returns the result in uppercase.
Previous: Check if Number is Multiple of 3 or 7.
NEXT : Check if String Starts with 'Java'.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.