JavaScript: Remove a character at the specified position of a given string and return the new string
JavaScript Basic: Exercise-22 with Solution
Remove Character at Specified Position in String
Write a JavaScript program to remove a character at the specified position in a given string and return the modified string.
This JavaScript program removes a character at a specified position in a given string and returns the modified string. It takes the position as input and removes the character at that position using string manipulation methods like substring() or slice(). Finally, it returns the modified string after removing the character at the specified position.
Visual Presentation:
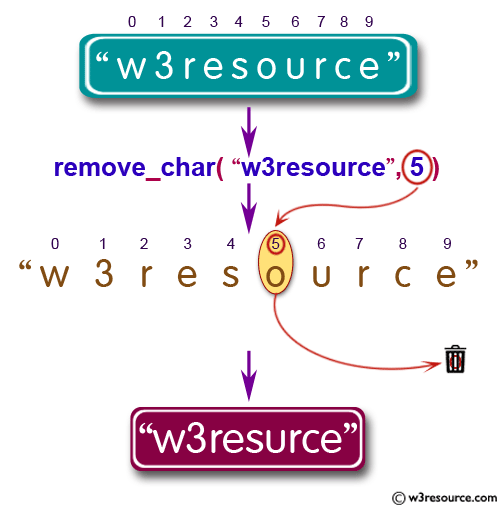
Sample Solution:
JavaScript Code:
// Define a function named remove_character that takes two parameters, str and char_pos
function remove_character(str, char_pos) {
// Extract the substring from the beginning of str up to (but not including) char_pos
part1 = str.substring(0, char_pos);
// Extract the substring from char_pos + 1 to the end of str
part2 = str.substring(char_pos + 1, str.length);
// Return the concatenation of part1 and part2, effectively removing the character at char_pos
return (part1 + part2);
}
// Log the result of calling the remove_character function with the arguments "Python" and 0 to the console
console.log(remove_character("Python", 0));
// Log the result of calling the remove_character function with the arguments "Python" and 3 to the console
console.log(remove_character("Python", 3));
// Log the result of calling the remove_character function with the arguments "Python" and 5 to the console
console.log(remove_character("Python", 5));
Output:
ython Pyton Pytho
Live Demo:
See the Pen JavaScript: characterposition: basic-ex-22 by w3resource (@w3resource) on CodePen.
Flowchart:
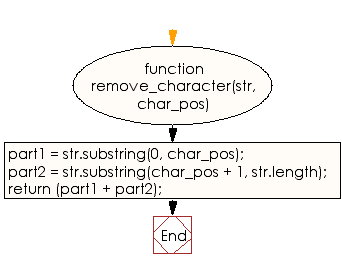
ES6 Version:
// Using ES6 arrow function syntax to define the remove_character function
const remove_character = (str, char_pos) => {
// Extract the substring from the beginning of str up to (but not including) char_pos
const part1 = str.substring(0, char_pos);
// Extract the substring from char_pos + 1 to the end of str
const part2 = str.substring(char_pos + 1, str.length);
// Return the concatenation of part1 and part2, effectively removing the character at char_pos
return part1 + part2;
};
// Log the result of calling the remove_character function with the arguments "Python" and 0 to the console
console.log(remove_character("Python", 0));
// Log the result of calling the remove_character function with the arguments "Python" and 3 to the console
console.log(remove_character("Python", 3));
// Log the result of calling the remove_character function with the arguments "Python" and 5 to the console
console.log(remove_character("Python", 5));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes the character at a user-specified index from a string, handling out-of-range indexes gracefully.
- Write a JavaScript program that removes characters at multiple specified positions in a given string.
- Write a JavaScript program that removes a character at a specified position and then inserts a dash in its place.
Go to:
PREV : Add 'Py' to Start of String if Not Present.
NEXT : Swap First and Last Characters in String.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.