JavaScript: Create a new string adding "Py" in front of a given string
JavaScript Basic: Exercise-21 with Solution
Add 'Py' to Start of String if Not Present
Write a JavaScript program to create another string by adding "Py" in front of a given string. If the given string begins with "Py" return the original string.
This JavaScript program creates a new string by adding "Py" in front of a given string. However, if the given string already begins with "Py", it returns the original string without any modification. It checks the initial characters of the given string to determine whether "Py" needs to be added or not.
Visual Presentation:
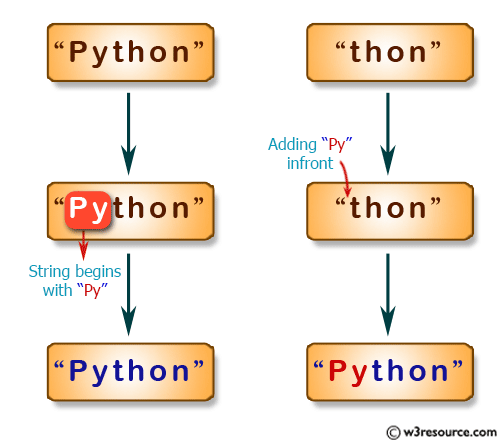
Sample Solution:
JavaScript Code:
// Define a function named string_check that takes a parameter str1
function string_check(str1) {
// Check if str1 is null, undefined, or starts with the substring 'Py'
if (str1 === null || str1 === undefined || str1.substring(0, 2) === 'Py') {
// If true, return str1
return str1;
}
// If false, prepend 'Py' to str1 and return the result
return "Py" + str1;
}
// Log the result of calling the string_check function with the argument "Python" to the console
console.log(string_check("Python"));
// Log the result of calling the string_check function with the argument "thon" to the console
console.log(string_check("thon"));
Output:
Python Python
Live Demo:
See the Pen JavaScript: Create new string: basic-ex-21 by w3resource (@w3resource) on CodePen.
Flowchart:
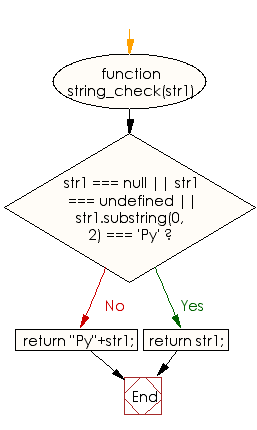
ES6 Version:
// Using ES6 arrow function syntax to define the string_check function
const string_check = (str1) => (str1 === null || str1 === undefined || str1.substring(0, 2) === 'Py' ? str1 : `Py${str1}`);
// Log the result of calling the string_check function with the argument "Python" to the console
console.log(string_check("Python"));
// Log the result of calling the string_check function with the argument "thon" to the console
console.log(string_check("thon"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that prepends 'Py' to a string if it doesn't already start with 'Py', ignoring case sensitivity.
- Write a JavaScript program that adds 'Py' to the beginning of a string only if the string length is even.
- Write a JavaScript program that checks if a string starts with 'py' (in any case) and adds it if missing, then returns the modified string.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check from two given integers, if one is positive and one is negative.
Next: JavaScript program to remove a character at the specified position of a given string and return the new string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.