JavaScript: Check two given integers, whether one is positive and another one is negative
JavaScript Basic: Exercise-20 with Solution
Check if One Integer is Positive and One is Negative
Write a JavaScript program to check two given integers whether one is positive and another one is negative.
This JavaScript program checks two given integers to determine if one is positive and the other is negative. It utilizes conditional statements and logical operators to evaluate whether one integer is greater than zero (positive) and the other is less than zero (negative), returning true if both conditions are met and false otherwise.
Visual Presentation:
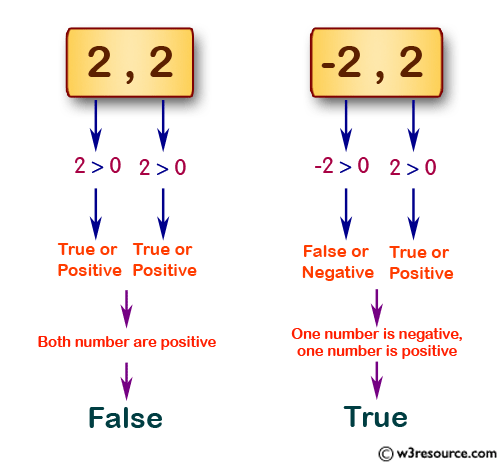
Sample Solution:
JavaScript Code:
Output:
false true true false
Live Demo:
Flowchart:
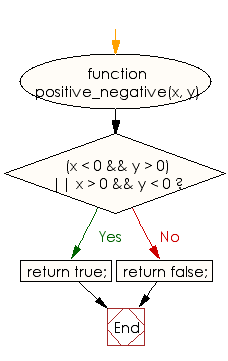
ES6 Version:
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if two given integers consist of one positive and one negative number.
- Write a JavaScript program that verifies if exactly one out of three integers is negative.
- Write a JavaScript program that determines if one number is positive and the other negative, and if their absolute values differ by more than 50.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check a given integer is within 20 of 100 or 400.
Next: JavaScript program to create a new string adding "Py" in front of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.