JavaScript: Compute the absolute difference between a specified number and 19
JavaScript Basic: Exercise-17 with Solution
Difference Between Number and 19 (Triple if >19)
Write a JavaScript program to compute the absolute difference between a specified number and 19. Returns triple the absolute difference if the specified number is greater than 19.
This JavaScript program computes the absolute difference between a specified number and 19. If the specified number is greater than 19, it returns triple the absolute difference. It utilizes conditional statements to check the given condition and perform the appropriate computation.
Sample Solution:
JavaScript Code:
// Define a function named diff_num that takes a parameter n
function diff_num(n) {
// Check if n is less than or equal to 19
if (n <= 19) {
// If true, return the difference between 19 and n
return (19 - n);
} else {
// If false, return three times the difference between n and 19
return (n - 19) * 3;
}
}
// Log the result of calling the diff_num function with the argument 12 to the console
console.log(diff_num(12));
// Log the result of calling the diff_num function with the argument 19 to the console
console.log(diff_num(19));
// Log the result of calling the diff_num function with the argument 22 to the console
console.log(diff_num(22));
Output:
7 0 9
Live Demo:
See the Pen JavaScript: Absolute difference- basic-ex-17 by w3resource (@w3resource) on CodePen.
Flowchart:
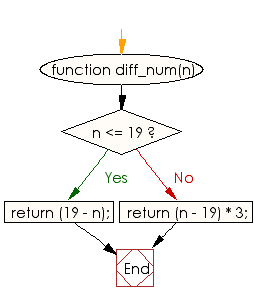
ES6 Version:
// Using ES6 arrow function syntax to define the diff_num function
const diff_num = (n) => {
// Check if n is less than or equal to 19
if (n <= 19) {
// If true, return the difference between 19 and n
return 19 - n;
} else {
// If false, return three times the difference between n and 19
return (n - 19) * 3;
}
};
// Log the result of calling the diff_num function with the argument 12 to the console
console.log(diff_num(12));
// Log the result of calling the diff_num function with the argument 19 to the console
console.log(diff_num(19));
// Log the result of calling the diff_num function with the argument 22 to the console
console.log(diff_num(22));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes the absolute difference between a given number and 19, returning triple the difference if the number exceeds 19 by at least 5.
- Write a JavaScript program that calculates the difference between a number and 19, doubling it if the number is less than 19.
- Write a JavaScript program that takes a user-provided number and returns triple the absolute difference from 19 if it is greater than 19, otherwise returns the simple difference.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to compute the sum of the two given integers.
Next: JavaScript program to check two given numbers and return true if one of the number is 50 or if their sum is 50.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.