JavaScript: Compute the sum of the two given integers
JavaScript Basic: Exercise-16 with Solution
Sum Two Integers (Triple if Equal)
Write a JavaScript program to compute the sum of the two given integers. If the two values are the same, then return triple their sum.
This JavaScript program calculates the sum of two given integers. If the two integers are the same, it returns triple their sum. It demonstrates conditional statements to determine whether the integers are equal and to perform the appropriate computation accordingly.
Visual Presentation:
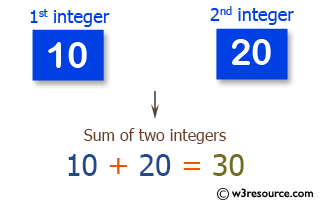
Sample Solution:
JavaScript Code:
// Define a function named sumTriple that takes two parameters, x and y
function sumTriple(x, y) {
// Check if x is equal to y
if (x == y) {
// If true, return three times the sum of x and y
return 3 * (x + y);
} else {
// If false, return the sum of x and y
return (x + y);
}
}
// Log the result of calling the sumTriple function with the arguments 10 and 20 to the console
console.log(sumTriple(10, 20));
// Log the result of calling the sumTriple function with the arguments 10 and 10 to the console
console.log(sumTriple(10, 10));
Output:
30 60
Live Demo:
See the Pen JavaScript: Sum of two integers- basic-ex-16 by w3resource (@w3resource) on CodePen.
Flowchart:
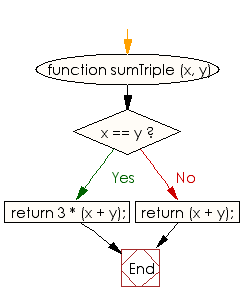
ES6 Version:
// Using ES6 arrow function syntax to define the sumTriple function
const sumTriple = (x, y) => {
// Check if x is equal to y
if (x == y) {
// If true, return three times the sum of x and y
return 3 * (x + y);
} else {
// If false, return the sum of x and y
return (x + y);
}
};
// Log the result of calling the sumTriple function with the arguments 10 and 20 to the console
console.log(sumTriple(10, 20));
// Log the result of calling the sumTriple function with the arguments 10 and 10 to the console
console.log(sumTriple(10, 10));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to get the difference between a given number
Next: JavaScript program to compute the absolute difference between a specified number and 19.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics