JavaScript: Swap pairs of adjacent digits of a given integer of even length
JavaScript Basic: Exercise-150 with Solution
Swap Adjacent Pairs in Even-Length Integer
Write a JavaScript program to swap pairs of adjacent digits of a given integer of even length.
Visual Presentation:
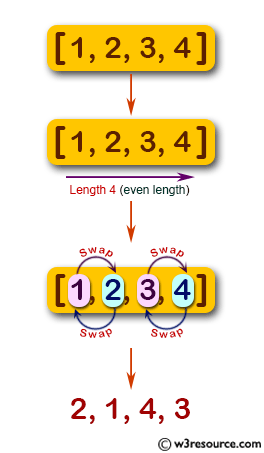
Sample Solution:
JavaScript Code:
/**
* Function to swap adjacent digits of a number
* @param {number} n - The input number
* @returns {(number|boolean)} - The number with adjacent digits swapped or `false` if the length is odd
*/
function swap_adjacent_digits(n) {
// Check if the length of the number is odd, return `false` in such cases
if (n.toString().length % 2 != 0) {
return false;
}
var result = 0,
x = 1;
// Loop until the number becomes zero
while (n != 0) {
// Get the last digit (dg1) and the second last digit (dg2)
var dg1 = n % 10,
dg2 = ((n - dg1) / 10) % 10;
// Swap dg1 and dg2, add to the result with appropriate position
result += x * (10 * dg1 + dg2);
// Remove the last two digits from the number
n = Math.floor(n / 100);
// Increment the multiplier x by 100
x *= 100;
}
return result; // Return the number with swapped adjacent digits
}
// Test cases
console.log(swap_adjacent_digits(15));
console.log(swap_adjacent_digits(1234));
console.log(swap_adjacent_digits(123456));
console.log(swap_adjacent_digits(12345));
Output:
51 2143 214365 false
Live Demo:
See the Pen javascript-basic-exercise-150 by w3resource (@w3resource) on CodePen.
Flowchart:
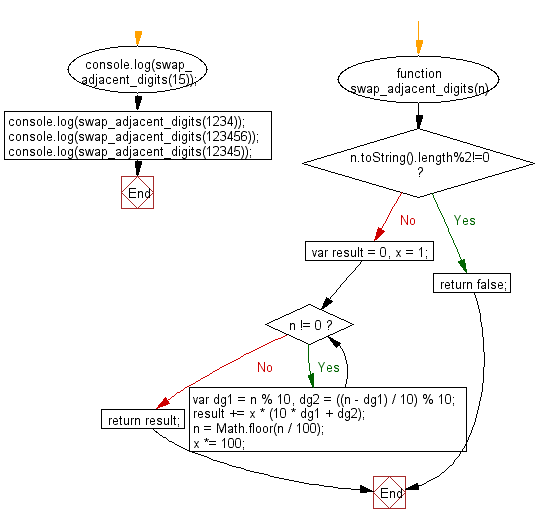
ES6 Version:
/**
* Function to swap adjacent digits of a number
* @param {number} n - The input number
* @returns {(number|boolean)} - The number with adjacent digits swapped or `false` if the length is odd
*/
const swap_adjacent_digits = n => {
// Check if the length of the number is odd, return `false` in such cases
if (n.toString().length % 2 !== 0) {
return false;
}
let result = 0,
x = 1;
// Loop until the number becomes zero
while (n !== 0) {
// Get the last digit (dg1) and the second last digit (dg2)
const dg1 = n % 10,
dg2 = ((n - dg1) / 10) % 10;
// Swap dg1 and dg2, add to the result with appropriate position
result += x * (10 * dg1 + dg2);
// Remove the last two digits from the number
n = Math.floor(n / 100);
// Increment the multiplier x by 100
x *= 100;
}
return result; // Return the number with swapped adjacent digits
}
// Test cases
console.log(swap_adjacent_digits(15)); // Output: false (length is odd)
console.log(swap_adjacent_digits(1234)); // Output: 2143
console.log(swap_adjacent_digits(123456)); // Output: 214365
console.log(swap_adjacent_digits(12345)); // Output: false (length is odd)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that swaps every two adjacent digits in a given even-length integer and returns the new integer.
- Write a JavaScript function that converts an integer to a string, swaps adjacent pairs of characters, and returns the modified number.
- Write a JavaScript program that validates an integer’s even digit length and performs pairwise digit swaps to reconstruct the integer.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to change the capitalization of all letters in a given string.
Next: Javascript Functions Exercises.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.