JavaScript: Swap two halves of a given array of integers of even length
JavaScript Basic: Exercise-148 with Solution
Swap Halves of Even-Length Array
Write a JavaScript program to swap two halves of a given array of integers of even length.
Visual Presentation:
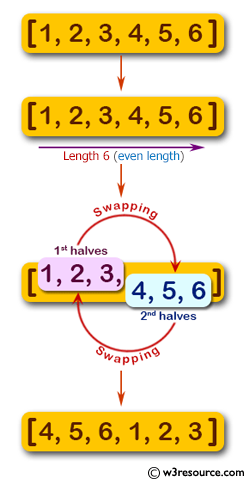
Sample Solution:
JavaScript Code:
/**
* Function to swap the halves of an array
* @param {Array} iarra - The input array
* @returns {(boolean|Array)} - Swapped array if even length, otherwise false
*/
function halv_array_swap(iarra) {
// Check if the array length is even
if ((iarra.length % 2) !== 0) {
return false; // Return false if the array length is odd
}
// Swap the halves of the array
for (var i = 0; i < iarra.length / 2; i++) {
var tmp = iarra[i]; // Temporary variable to store the current element
iarra[i] = iarra[i + iarra.length / 2]; // Swap the first half with the second half
iarra[i + iarra.length / 2] = tmp; // Swap the second half with the first half
}
return iarra; // Return the swapped array
}
// Test cases
console.log(halv_array_swap([1, 2, 3, 4, 5, 6])); // Output: [4, 5, 6, 1, 2, 3]
console.log(halv_array_swap([1, 2, 3, 4, 5, 6, 7])); // Output: false
Output:
[4,5,6,1,2,3] false
Live Demo:
See the Pen javascript-basic-exercise-148 by w3resource (@w3resource) on CodePen.
Flowchart:
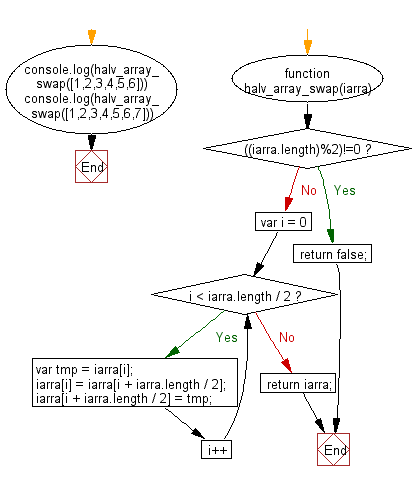
ES6 Version:
/**
* Function to swap the halves of an array
* @param {Array} iarra - The input array
* @returns {(boolean|Array)} - Swapped array if even length, otherwise false
*/
const halv_array_swap = (iarra) => {
// Check if the array length is even
if ((iarra.length % 2) !== 0) {
return false; // Return false if the array length is odd
}
// Swap the halves of the array
for (let i = 0; i < iarra.length / 2; i++) {
// Use destructuring assignment for swapping elements
[iarra[i], iarra[i + iarra.length / 2]] = [iarra[i + iarra.length / 2], iarra[i]];
}
return iarra; // Return the swapped array
};
// Test cases
console.log(halv_array_swap([1, 2, 3, 4, 5, 6])); // Output: [4, 5, 6, 1, 2, 3]
console.log(halv_array_swap([1, 2, 3, 4, 5, 6, 7])); // Output: false
For more Practice: Solve these Related Problems:
- Write a JavaScript program that splits an even-length array into two halves and swaps their positions.
- Write a JavaScript function that divides an array into equal halves and returns a new array with the halves exchanged.
- Write a JavaScript program that validates an array’s even length and then performs an in-place swap of its first and second halves.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to compute the sum of all digits that occur in a given string.
Next: JavaScript program to change the capitalization of all letters in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.