JavaScript: Compute the sum of all digits that occur in a given string
JavaScript Basic: Exercise-147 with Solution
Sum of Digits in String
Write a JavaScript program to compute the sum of all the digits that occur in a given string.
Visual Presentation:
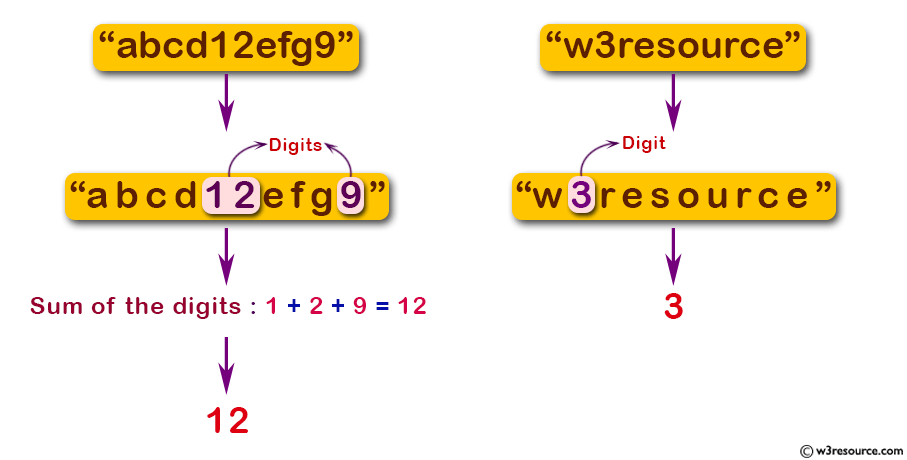
Sample Solution:
JavaScript Code:
/**
* Function to calculate the sum of digits from a string
* @param {string} dstr - The string containing alphanumeric characters
* @returns {number} - The sum of digits found in the string
*/
function sum_digits_from_string(dstr) {
var dsum = 0; // Variable to hold the sum of digits
for (var i = 0; i < dstr.length; i++) {
// Check if the character is a digit, then convert and add it to 'dsum'
if (/[0-9]/.test(dstr[i])) dsum += parseInt(dstr[i]);
}
return dsum; // Return the sum of digits from the string
}
// Display the sum of digits for different strings
console.log(sum_digits_from_string("abcd12efg9")); // Output: 12
console.log(sum_digits_from_string("w3resource")); // Output: 3
Output:
12 3
Live Demo:
See the Pen javascript-basic-exercise-147 by w3resource (@w3resource) on CodePen.
Flowchart:
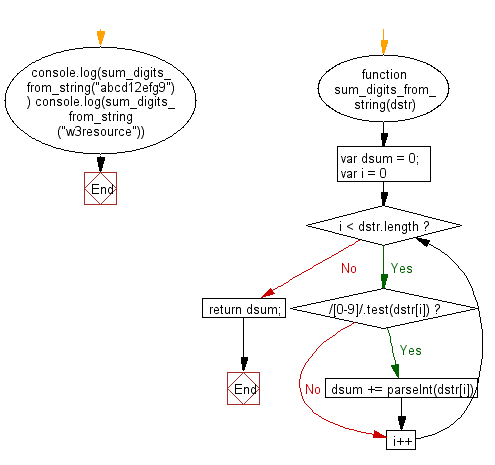
ES6 Version:
/**
* Function to calculate the sum of digits from a string
* @param {string} dstr - The string containing alphanumeric characters
* @returns {number} - The sum of digits found in the string
*/
const sum_digits_from_string = (dstr) => {
let dsum = 0; // Variable to hold the sum of digits
for (let i = 0; i < dstr.length; i++) {
// Check if the character is a digit, then convert and add it to 'dsum'
if (/[0-9]/.test(dstr[i])) dsum += parseInt(dstr[i]);
}
return dsum; // Return the sum of digits from the string
};
// Display the sum of digits for different strings
console.log(sum_digits_from_string("abcd12efg9")); // Output: 12
console.log(sum_digits_from_string("w3resource")); // Output: 3
For more Practice: Solve these Related Problems:
- Write a JavaScript program that extracts all digit characters from a string and computes their total sum.
- Write a JavaScript function that iterates over a string, converts each digit character to a number, and returns the cumulative sum.
- Write a JavaScript program that filters out non-digit characters from a string and calculates the sum of all numerical digits found.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to compute the sum of cubes of all integer from 1 to a given integer.
Next: JavaScript program to swap two halves of a given array of integers of even length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.