JavaScript: Compute the sum of cubes of all integer from 1 to an given integer
JavaScript Basic: Exercise-146 with Solution
Sum of Cubes from 1 to n
Write a JavaScript program to compute the sum of cubes of all integers from 1 to a given integer.
Sample Solution:
JavaScript Code:
/**
* Function to calculate the sum of cubes up to a given number 'n'
* @param {number} n - The number up to which the sum of cubes needs to be calculated
* @returns {number} - The sum of cubes up to the given number
*/
function sum_Of_Cubes(n) {
var sumn = 0; // Variable to hold the sum of cubes
// Loop to calculate the sum of cubes up to the number 'n'
for (var i = 1; i <= n; i++) {
sumn += Math.pow(i, 3); // Add the cube of 'i' to the sum
}
return sumn; // Return the total sum of cubes
}
// Display the sum of cubes for different values of 'n'
console.log(sum_Of_Cubes(3)); // Output: 36
console.log(sum_Of_Cubes(4)); // Output: 100
Output:
36 100
Live Demo:
See the Pen javascript-basic-exercise-146 by w3resource (@w3resource) on CodePen.
Flowchart:
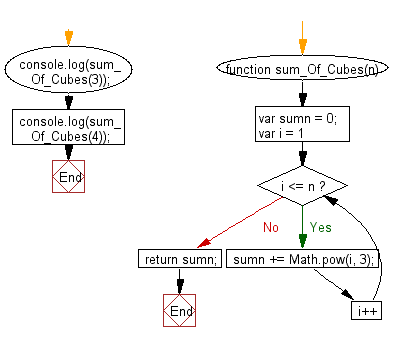
ES6 Version:
/**
* Function to calculate the sum of cubes up to a given number 'n'
* @param {number} n - The number up to which the sum of cubes needs to be calculated
* @returns {number} - The sum of cubes up to the given number
*/
const sum_Of_Cubes = (n) => {
let sumn = 0; // Variable to hold the sum of cubes
// Loop to calculate the sum of cubes up to the number 'n'
for (let i = 1; i <= n; i++) {
sumn += Math.pow(i, 3); // Add the cube of 'i' to the sum
}
return sumn; // Return the total sum of cubes
};
// Display the sum of cubes for different values of 'n'
console.log(sum_Of_Cubes(3)); // Output: 36
console.log(sum_Of_Cubes(4)); // Output: 100
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes the sum of cubes of all integers from 1 to n using an iterative approach.
- Write a JavaScript function that calculates the cube of each number in the range 1 to n and returns the total sum.
- Write a JavaScript program that returns the sum of cubes from 1 to n and validates that n is a positive integer.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the maximum integer n such that 1 + 2 + ... + n <= a given integer.
Next: JavaScript program to compute the sum of all digits that occur in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.