JavaScript: Find the maximum integer n
JavaScript Basic: Exercise-145 with Solution
Max n for 1+2+...+n ≤ Value
Write a JavaScript program to find the maximum integer n such that 1 + 2 + ... + n <= a given integer.
Sample Solution:
JavaScript Code:
/**
* Function to find the sum of natural numbers up to a given value
* @param {number} val - The value up to which the sum of natural numbers needs to be calculated
* @returns {number} - The number of terms in the sum sequence
*/
function sumn(val) {
var sn = 0; // Initialize the sum of natural numbers
var i = 0; // Initialize the index to 0
// Loop to calculate the sum of natural numbers up to a certain limit
while (sn <= val) {
sn += i++; // Incrementally add the value of 'i' to the sum and increment 'i'
}
return i - 2; // Return the number of terms in the sum sequence (subtracting 2 to account for the extra iteration)
}
// Display the number of terms for different values
console.log(sumn(11)); // Output: 4
console.log(sumn(15)); // Output: 5
Output:
4 5
Live Demo:
See the Pen javascript-basic-exercise-145 by w3resource (@w3resource) on CodePen.
Flowchart:
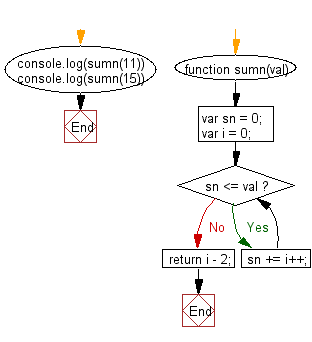
ES6 Version:
/**
* Function to find the sum of natural numbers up to a given value
* @param {number} val - The value up to which the sum of natural numbers needs to be calculated
* @returns {number} - The number of terms in the sum sequence
*/
const sumn = (val) => {
let sn = 0; // Initialize the sum of natural numbers
let i = 0; // Initialize the index to 0
// Loop to calculate the sum of natural numbers up to a certain limit
while (sn <= val) {
sn += i++; // Incrementally add the value of 'i' to the sum and increment 'i'
}
return i - 2; // Return the number of terms in the sum sequence (subtracting 2 to account for the extra iteration)
};
// Display the number of terms for different values
console.log(sumn(11)); // Output: 4
console.log(sumn(15)); // Output: 5
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to sort the strings of a given array of strings in the order of increasing lengths.
Next: JavaScript program to compute the sum of cubes of all integer from 1 to a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics